How to Convert a String to a Number in SQL | Explained
In SQL, converting strings to numbers can be done using the CAST and CONVERT functions, such as SELECT CAST(’42’ AS INT) AS ConvertedValue. The TRY_CAST and TRY_CONVERT functions handle errors gracefully, returning NULL for failed conversions.
Numeric operations, like addition (SELECT ’20’ + 10 AS ConvertedValue), or conditional checks using CASE statements, offer alternative methods. Dealing with non-numeric characters can be addressed using functions like ISNUMERIC or additional checks within CASE statements.
I have covered you from every side, let’s unfold.
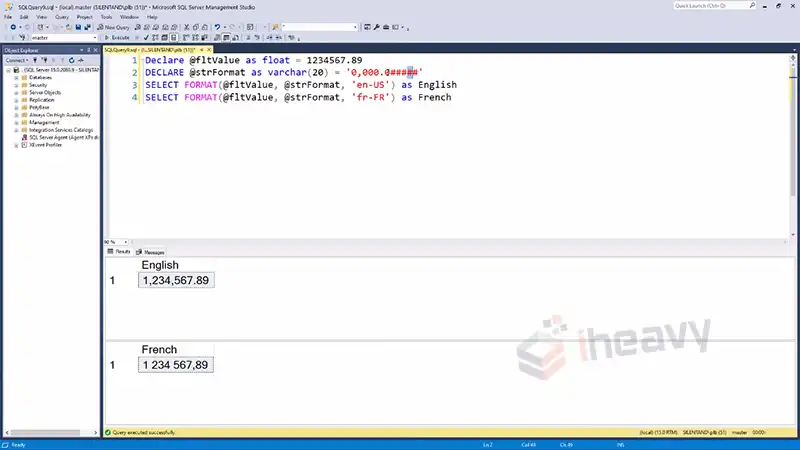
Data Types in SQL
Strings, represented by VARCHAR or CHAR, store textual data, while numeric data comes in various forms like INTEGER, DECIMAL, FLOAT, and more. The challenge arises when you need to convert a string value into a numeric type to perform arithmetic operations or comparisons accurately.
Converting String to Number in SQL
Here’s an overview of common ways to achieve this:
Using SQL Conversion Functions
1. CAST and CONVERT Functions
The `CAST` and `CONVERT` functions in SQL are powerful tools for data type conversion. They allow you to explicitly specify the target data type for a string value. For instance:
SELECT CAST('42' AS INT) AS ConvertedValue;
This query converts the string ’42’ into an integer using the `CAST` function. Similarly, the `CONVERT` function achieves the same result:
SELECT CONVERT(INT, '42') AS ConvertedValue;
2. TRY_CAST and TRY_CONVERT
These functions are variations of `CAST` and `CONVERT` that handle potential errors more gracefully. If the conversion fails, they return a NULL value instead of throwing an error, which can be helpful when dealing with uncertain or varied data:
SELECT TRY_CAST('abc' AS INT) AS ConvertedValue; -- Returns NULL
Using Numeric Functions to Convert
SQL also provides numeric functions that can indirectly convert strings to numbers:
1. Using Arithmetic Operations
Simple arithmetic operations can coerce strings into numeric values during execution:
SELECT '20' + 10 AS ConvertedValue; -- Returns 30
Here, SQL implicitly converts the string ’20’ to a numeric value for addition.
2. Using CASE Statements
You can employ `CASE` statements to conditionally convert strings based on specific criteria:
SELECT
CASE
WHEN ISNUMERIC('123') = 1 THEN CAST('123' AS INT)
ELSE NULL
END AS ConvertedValue;
This query checks if the string ‘123’ is numeric and converts it to an integer if true.
Dealing with Non-Numeric Values
Converting strings to numbers becomes challenging when dealing with non-numeric characters. Functions like `ISNUMERIC` or additional checks within `CASE` statements can help identify and handle non-convertible strings appropriately.
Frequently Asked Questions
Does converting strings to numbers affect performance in SQL queries?
Generally, converting strings to numbers in SQL queries has minimal impact on performance. However, extensive use of conversion functions or handling large volumes of data could marginally impact query execution time.
Are there differences in syntax for string-to-number conversion across various SQL databases?
Yes, there might be variations in syntax. For instance, PostgreSQL uses the:: operator for casting, while Oracle has its TO_NUMBER function. Always refer to the specific database documentation for accurate syntax.
What precautions should I take when converting strings to numbers in SQL?
Validate the string data to ensure it only contains valid numeric values. Test conversions on sample data before applying to larger datasets to avoid unexpected results or errors.
Where can I find more information about string-to-number conversion in SQL for my specific database?
Refer to the official documentation of your SQL database system for comprehensive information, supported functions, and best practices related to string-to-number conversions.
Conclusion
Mastering the art of converting strings to numbers in SQL is crucial for efficient data manipulation and analysis. Whether using explicit conversion functions like `CAST` and `CONVERT`, leveraging numeric operations, or employing conditional statements, SQL offers a variety of tools to handle these conversions.