Convert Varchar to Float in SQL | 3 Methods to Crack It
Ensuring data integrity and consistency is held important. However, data isn’t always stored in the most compatible formats, leading to the need for conversions. One common transformation is converting varchar data types to float in SQL databases.
To state simply, to convert a varchar column to float in SQL, you can use the CAST or CONVERT functions. But that’s just the tip of the iceberg. This article provides a comprehensive guide on executing this conversion.
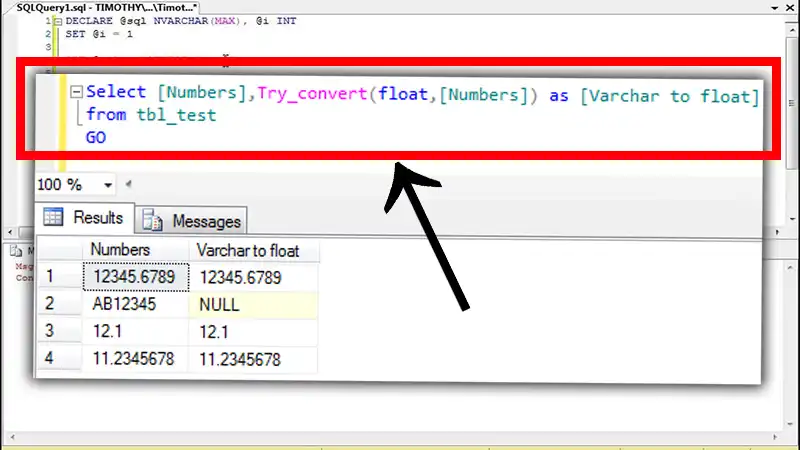
Varchar and Float Data Types
Before diving into conversions, it’s crucial to comprehend the data types involved. Varchar represents variable-length character strings, capable of storing alphanumeric characters and symbols. On the other hand, float is a numeric data type designed to store decimal numbers with varying precision.
How to Convert Varchar to Float in SQL?
Converting varchar to float in SQL involves several methods to ensure accurate and error-free transformations. Here’s a step-by-step guide on how to perform this conversion:
Method 1: Using CAST or CONVERT Functions
SQL offers the `CAST` and `CONVERT` functions to convert data types. Follow these steps:
Step 1: Identify the Varchar Column
Understand which varchar column needs conversion. Let’s assume you have a column named `varchar_column` in a table named `your_table`.
Step 2: Use the CAST Function
Execute a SQL query using the `CAST` function to convert varchar to float:
SELECT CAST(varchar_column AS FLOAT) AS converted_value
FROM your_table;
This query will return the `varchar_column` values converted to the float data type in a new column named `converted_value`.
Method 2: Data Cleansing and Conversion
Sometimes, varchar columns might contain non-numeric characters or inconsistencies. Cleaning the data beforehand is crucial to ensure successful conversion:
Step 1: Clean the Data
Ensure the varchar data is cleaned by removing non-numeric characters, handling null values, and trimming extra spaces.
UPDATE your_table
SET varchar_column = REPLACE(varchar_column, ',', '.') -- If dealing with decimal points
WHERE ISNUMERIC(REPLACE(varchar_column, ',', '.')) = 1;
This example replaces commas with periods to handle decimal points and updates the column if the data is numeric.
Step 2: Convert to Float
After cleaning, perform the conversion using the `CAST` function:
ALTER TABLE your_table
ALTER COLUMN varchar_column FLOAT;
This alters the data type of the `varchar_column` from varchar to float in the `your_table`.
Method 3: Validation and Error Handling
Implement validation checks to handle exceptions and ensure data integrity:
Step 1: Validate Data
Use validation checks to identify and handle invalid entries before conversion:
SELECT varchar_column
FROM your_table
WHERE TRY_CAST(varchar_column AS FLOAT) IS NULL;
This query identifies entries that cannot be converted to float, allowing you to address them accordingly.
Step 2: Handle Exceptions
Implement error handling mechanisms, such as try-catch blocks, to manage conversion failures gracefully:
BEGIN TRY
ALTER TABLE your_table
ALTER COLUMN varchar_column FLOAT;
END TRY
BEGIN CATCH
-- Handle conversion errors here
END CATCH;
Challenges and Considerations
Converting varchar to float isn’t always straightforward due to potential data inconsistencies. Here are key challenges and considerations:
1. Data Integrity: Varchar fields may contain non-numeric characters, leading to conversion errors or unexpected results. Cleaning the data before conversion is crucial.
2. Precision Loss: Float data types have limited precision. Converting from varchar to float may result in rounding errors or loss of precision, affecting calculations.
3. Error Handling: Invalid data entries can cause conversion failures. Implementing error-handling mechanisms is essential to manage exceptions gracefully.
Best Practices
1. Backup Data: Always back up your data before performing conversions to prevent irreversible data loss.
2. Test Thoroughly: Execute conversions on a subset of data or in a testing environment to validate accuracy before applying changes to the entire dataset.
3. Handle Exceptions: Implement error handling mechanisms, such as try-catch blocks, to manage conversion failures gracefully.
4. Consider Alternative Approaches: Depending on the data and requirements, consider alternative data types or normalization techniques to minimize conversion complexities.
Frequently Asked Questions
Why would I need to convert varchar to float in SQL?
Converting varchar to float is necessary when numeric values are stored as strings (varchar) but need to be used for mathematical calculations or to adhere to a consistent data type across the database.
Can converting varchar to float cause data loss or change in values?
Yes, there might be precision loss or rounding errors, especially if the varchar data has a higher precision than what the float type allows.
What should I do if the conversion fails due to errors or exceptions?
Implement error handling mechanisms such as try-catch blocks to manage conversion failures gracefully. Backing up data before conversion is also advisable.
Conclusion
Maintaining data integrity throughout the process is imperative to ensure accurate results. Specially, it’s essential to ensure data cleanliness by handling non-numeric characters and potential null values. Additionally, implementing validation checks and error handling mechanisms helps maintain data integrity during the conversion process.