Cannot Convert From Byte to JAVA. SQL. TIMESTAMP | Explained
The conversion challenge between byte data and Java’s SQL TIMESTAMP type poses a significant hurdle in programming. Converting raw byte information into a recognizable TIMESTAMP format within Java requires precision and understanding of data representation.
This article aims to dissect the complexities behind this conversion issue and potential solutions to bridge the gap between byte data and the TIMESTAMP type in Java’s SQL environment.
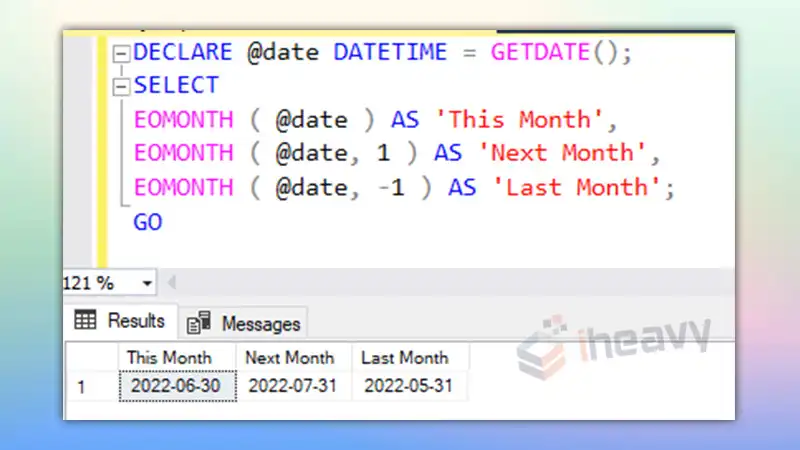
The Byte Type
Bytes are fundamental units of digital information, representing a sequence of eight bits. In Java, the byte data type is an 8-bit signed two’s complement integer. It’s commonly used when dealing with raw binary data or when memory efficiency is a concern. However, its limitation lies in representing a relatively small range of values from -128 to 127.
The Java SQL Timestamp Type
On the other hand, a Java SQL Timestamp is a more specialized data type used to store date and time information. It extends the functionality of the `java.util.Date` class and includes the ability to hold the SQL `TIMESTAMP` fractional seconds value. This data type is commonly employed in database operations where precise timestamp information is necessary.
The Conversion Challenge
The challenges in directly converting from a byte to a Java SQL Timestamp stem from the disparate nature of these representations:
Semantic Gap
Bytes are essentially raw data without inherent meaning. The interpretation of bytes to extract temporal information requires a predefined structure or encoding scheme. Converting these bytes into a meaningful Timestamp involves understanding the byte sequence’s encoding format or structure.
Data Loss or Misinterpretation
When attempting direct conversion, there’s a risk of losing information or misinterpreting the byte sequence. If the byte sequence doesn’t adhere to an expected format or if its representation is ambiguous, extracting accurate temporal data becomes challenging.
Contextual Understanding
Successful conversion often demands prior knowledge or contextual information about how the bytes were created or encoded. Without this understanding, interpreting bytes as a Timestamp becomes significantly more challenging.
Solving the Conversion Issue
Converting byte data to Java’s SQL Timestamp type involves a few steps, especially considering the different data formats and representations. Here’s a breakdown:
Byte to Java Conversion
1. Understanding Byte Data: Bytes represent raw binary data and could signify various types of information.
2. Convert Byte Array to Java Object: If your byte data represents a timestamp, you’ll need to convert it into a recognizable format for Java.
Handling Timestamps in Java
1. Using `java.sql.Timestamp`: This class is used to work with SQL TIMESTAMP type and represents a specific instant in time.
2. Conversion from Byte to Timestamp:
– Parse or extract the relevant bytes from your byte data that signify the timestamp.
– Use the constructor `Timestamp(long time)` or `Timestamp(String s)` to create a Timestamp object from the extracted bytes.
– If the byte data represents a Unix timestamp (milliseconds since January 1, 1970, 00:00:00 GMT), use `new Timestamp(long milliseconds)`.
Sample Java Code
Here’s a simple example demonstrating the conversion:
// Assuming byteData is your byte array representing a timestamp
byte[] byteData = // your byte array here;
// Assuming byteData contains 8 bytes representing a long value (timestamp in milliseconds)
// Convert the byte array to a long value
ByteBuffer buffer = ByteBuffer.wrap(byteData);
long timestampInMillis = buffer.getLong();
// Create a Timestamp object using the extracted milliseconds
Timestamp timestamp = new Timestamp(timestampInMillis);
// You can now work with the 'timestamp' object as needed
Important Points
– Ensure the byte data is formatted consistently and aligns with the intended timestamp representation (e.g., milliseconds since epoch).
– Handling endianness: Consider the byte order (little-endian or big-endian) if your byte data has a specific byte order.
– Verify the size and format of the byte array. For instance, timestamps could be represented using various byte lengths (e.g., 4 bytes for seconds or 8 bytes for milliseconds).
Always ensure accuracy and handle exceptions or edge cases that may arise during conversion. This sample code assumes the byte array represents a long value (8 bytes) for the timestamp. Adjust the logic based on the actual format and structure of your byte data.
Best Practices
1. Data Interpretation: Understand how the byte data encodes the timestamp information before attempting the conversion.
2. Error Handling: Account for potential errors during parsing or interpretation of byte data.
3. Testing: Thoroughly test the conversion process with various byte inputs to ensure accuracy and reliability.
Frequently Asked Questions
What precautions should I take when handling byte data for TIMESTAMP conversion?
Ensure consistent data formats, handle exceptions diligently, validate byte data against the expected TIMESTAMP format, and thoroughly test the conversion process with various data samples to ensure accuracy.
What are the common causes of conversion errors between byte and TIMESTAMP in Java?
Errors can stem from mismatched byte lengths for timestamp representation, differing byte order (little-endian vs. big-endian), incorrect interpretation of byte data as a timestamp, or discrepancies in the encoding/decoding process.
Conclusion
The inability to directly convert from a byte to a Java SQL Timestamp stems from their inherent differences in representation and purpose. Overcoming this challenge involves decoding the byte data and constructing the Timestamp object using the extracted information.