Convert JSON To Table In SQL Server Without OPENJSON | A Comprehensive Guide
If you want to convert JSON data to a table in SQL Server without using the OPENJSON function, you can use the JSON_VALUE and JSON_QUERY functions to extract specific values and arrays from JSON, respectively. However, keep in mind that this method is not as versatile as OPENJSON and is more suitable for simple JSON structures.
I have set an example of how you can achieve this easily.
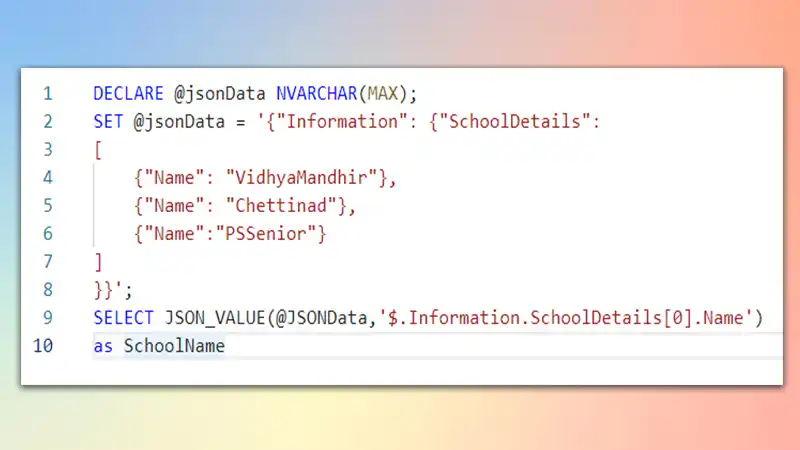
Conversion of JSON To Table In SQL Server Without OPENJSON
Assuming you have a table named YourTable with a column JsonColumn containing JSON data, and the JSON structure is simple (e.g., key-value pairs):
CREATE TABLE YourTable (
ID INT PRIMARY KEY,
JsonColumn NVARCHAR(MAX)
);
INSERT INTO YourTable (ID, JsonColumn)
VALUES
(1, '{"name": "John", "age": 30, "city": "New York"}'),
(2, '{"name": "Alice", "age": 25, "city": "Los Angeles"}');
You can then create a new table or use a temporary table to store the extracted values:
CREATE TABLE ExtractedData (
ID INT PRIMARY KEY,
Name NVARCHAR(50),
Age INT,
City NVARCHAR(50)
);
INSERT INTO ExtractedData (ID, Name, Age, City)
SELECT
ID,
JSON_VALUE(JsonColumn, '$.name') AS Name,
TRY_CAST(JSON_VALUE(JsonColumn, '$.age') AS INT) AS Age,
JSON_VALUE(JsonColumn, '$.city') AS City
FROM YourTable;
In this example:
- JSON_VALUE(JsonColumn, ‘$.name’): Extracts the value associated with the key “name” from the JSON.
- TRY_CAST(JSON_VALUE(JsonColumn, ‘$.age’) AS INT): Attempts to cast the value associated with the key “age” to an integer.
- JSON_VALUE(JsonColumn, ‘$.city’): Extracts the value associated with the key “city” from the JSON.
Frequently Asked Questions
1. Can I use JSON functions to extract values directly into a new table without OPENJSON?
Answer: Yes, you can use functions like JSON_VALUE and JSON_QUERY to extract specific values or objects from JSON and insert them directly into a new table. While not as versatile as OPENJSON, these functions are useful for simple JSON structures with key-value pairs.
2. How can I handle nested JSON structures without OPENJSON?
Answer: Handling nested structures without OPENJSON can be challenging, as the direct extraction functions work best for flat JSON structures. In cases of nested JSON, you may need to use a combination of JSON_VALUE and JSON_QUERY functions along with additional SQL logic to parse and flatten the nested data into a suitable tabular format.
3. Are there performance considerations when not using OPENJSON for JSON to table conversion?
Answer: Yes, performance considerations depend on the complexity of your JSON data. For simple structures, using direct extraction functions might be efficient. However, for complex or large datasets, OPENJSON can offer better performance as it allows more flexible handling of JSON arrays and objects. Always consider your specific use case and test the performance of different approaches to determine the most suitable method for your scenario.
Concluding Remarks
You should note that this approach assumes a fixed structure in the JSON data and may not be suitable for more complex JSON structures. If your JSON data has nested arrays or more complex hierarchies, using OPENJSON might be a more appropriate solution.