How to Check if an Element is Visible in the Viewport using JavaScript
Determining whether an element is currently visible within the user’s viewport is a common requirement in web development, especially for implementing features such as lazy loading of images or triggering animations as elements come into view. In this article, we’ll explore different methods in JavaScript to check if an element is visible within the viewport and discuss their implementation.
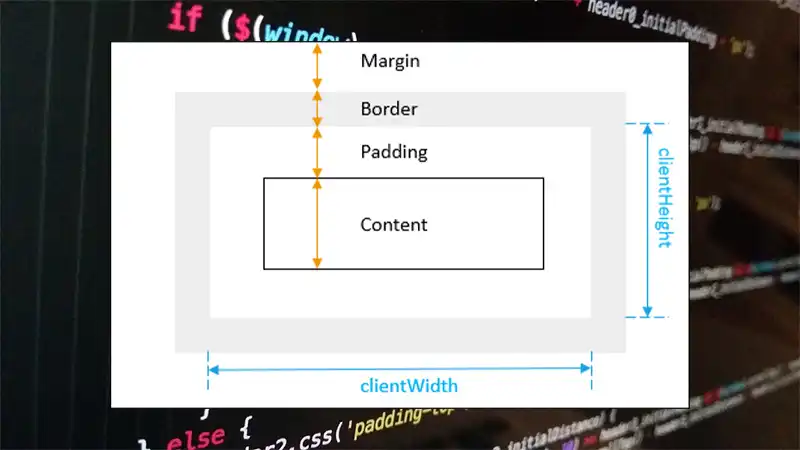
Using getBoundingClientRect() Method
The getBoundingClientRect() method returns the size of an element and its position relative to the viewport. By comparing the element’s position and dimensions with the viewport dimensions, we can determine if the element is currently visible. Here’s how you can implement it:
function isElementInViewport(el) {
var rect = el.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
);
}
Example usage
This function checks if all four sides of the element’s bounding rectangle are within the viewport boundaries.
var element = document.getElementById('myElement');
if (isElementInViewport(element)) {
console.log('Element is visible in the viewport');
} else {
console.log('Element is not visible in the viewport');
}
Using Intersection Observer API
The Intersection Observer API provides a more efficient way to detect when an element enters or exits the viewport. It allows you to asynchronously observe changes in the intersection between a target element and its containing ancestor or the viewport. Here’s how you can use it:
var observer = new IntersectionObserver(function(entries) {
entries.forEach(function(entry) {
if (entry.isIntersecting) {
console.log('Element is visible in the viewport');
} else {
console.log('Element is not visible in the viewport');
}
});
});
Example usage
With the Intersection Observer API, you can specify a callback function to be executed whenever the visibility of the observed element changes.
var element = document.getElementById('myElement');
observer.observe(element);
Frequently Asked Questions (FAQ)
Can I use CSS pseudo-classes like :visible to check if an element is visible?
CSS pseudo-classes like :visible only apply to elements that are visible according to the CSS rendering rules, not necessarily whether they are within the viewport. JavaScript methods are more suitable for viewport visibility checks.
Are there performance considerations when using these methods?
Both getBoundingClientRect() and Intersection Observer API are efficient and have minimal performance impact. However, it’s essential to use them judiciously, especially when observing multiple elements simultaneously.
Can I customize the threshold for intersection detection with the Intersection Observer API?
Yes, you can specify a threshold value (between 0 and 1) to control when the intersection callback is triggered. This allows you to define custom conditions for element visibility.
Conclusion
Detecting whether an element is visible in the viewport using JavaScript is a valuable technique for implementing various web functionalities. Whether you choose to use the getBoundingClientRect() method for precise calculations or leverage the Intersection Observer API for efficient observation, JavaScript provides flexible solutions to cater to your requirements. By mastering these methods, you can create engaging and interactive web experiences for users.