How to Compare Two Strings in Oracle SQL Query | A Comprehensive Guide
In Oracle SQL, comparing strings is a common operation, whether it’s for searching, sorting, or performing data analysis. Understanding the various methods available for string comparison is crucial for efficient query development.
This article will explore different techniques for comparing two strings in Oracle SQL queries.
What Is Oracle SQL Query?
Oracle SQL, or Structured Query Language, is a powerful domain-specific language used for managing and manipulating relational databases in Oracle Database Management System (DBMS).
SQL queries are commands that enable users to interact with the database, performing tasks like retrieving, inserting, updating, and deleting data.
These queries follow a standardized syntax and allow users to communicate with the Oracle database to retrieve specific information or perform various operations on the stored data. In essence, Oracle SQL queries serve as a means to interact with and extract meaningful insights from the underlying database.
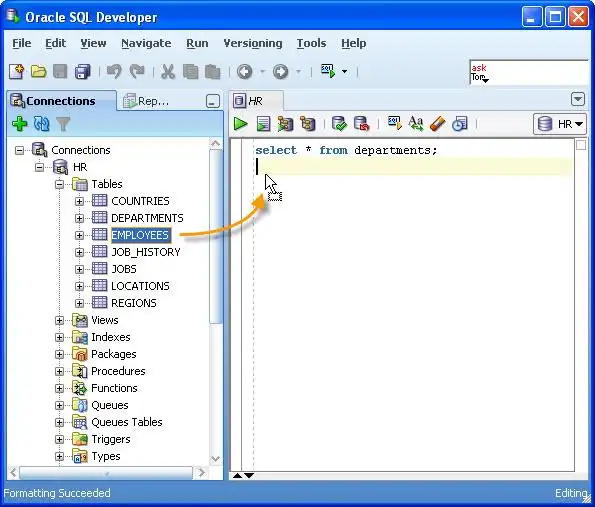
Steps to Compare Two Strings in Oracle SQL Query
1. Using the EQUALS (=) Operator
The most straightforward method is to use the equals (=) operator. This operator compares two strings character by character and returns true if they are an exact match. However, keep in mind that string comparison is case-sensitive in Oracle SQL, so “John” and “john” would be considered different.
SQL Command-
SELECT * FROM employees WHERE employee_name = 'John';
2. Case-Insensitive Comparison
If you need a case-insensitive comparison, you can use the `UPPER` or `LOWER` functions to convert both strings to either uppercase or lowercase before comparing.
SQL Command-
SELECT * FROM employees WHERE UPPER(employee_name) = UPPER('John');
3. LIKE Operator for Partial Matches
The `LIKE` operator is useful when you want to find strings that match a specified pattern. You can use wildcard characters such as ‘%’ to represent any sequence of characters.
SQL Command-
SELECT * FROM products WHERE product_name LIKE 'App%';
4. Pattern Matching with REGEXP_LIKE
For more advanced pattern matching, you can use the `REGEXP_LIKE` function, which allows the use of regular expressions. This is particularly helpful when you need to match strings based on complex patterns.
SQL Command-
SELECT * FROM customers WHERE REGEXP_LIKE(email, '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$');
5. STRING Comparison Functions
Oracle provides several string comparison functions like `STRCMP`, `STRCOLL`, and `NLSSORT`. These functions offer more advanced comparison options, including linguistic sorting based on the specified language.
SQL Command-
SELECT * FROM employees ORDER BY NLSSORT(employee_name, 'NLS_SORT = BINARY_AI');
6. Soundex and UTL_MATCH
Oracle offers the `SOUNDEX` function, which returns a phonetic representation of a string. Additionally, the `UTL_MATCH` package provides functions like `EDIT_DISTANCE` for calculating the edit distance between two strings.
SQL Command-
SELECT * FROM customers WHERE SOUNDEX(last_name) = SOUNDEX('Smith');
Troubleshooting Common Issues in String Comparison Queries
String comparison queries in Oracle SQL can sometimes present challenges that may lead to unexpected results or errors. Addressing these issues requires a combination of understanding the intricacies of string comparison and employing effective troubleshooting techniques.
1. Case-Sensitivity Problems
Issue
String comparison in Oracle SQL is case-sensitive by default, which may lead to inaccurate results when comparing strings with different cases.
Solution
Utilize the `UPPER` or `LOWER` functions to standardize the case for both compared strings. This ensures a case-insensitive comparison.
SQL Command-
SELECT * FROM employees WHERE UPPER(employee_name) = UPPER('John');
2. Handling NULL Values
Issue
Dealing with NULL values in string columns can affect the outcome of comparison queries.
Solution
Use the `NVL` function to replace NULL values with a specified default value before performing the comparison.
SQL Command-
SELECT * FROM customers WHERE NVL(email, 'N/A') = 'john@example.com';
3. Incorrect Use of Wildcards in LIKE Operator
Issue
Improper use of wildcard characters in the `LIKE` operator can result in unexpected matches or misses.
Solution
Ensure the correct placement of `%` or `_` wildcards based on the desired pattern. Test the query with sample data to validate the results.
SQL Command-
SELECT * FROM products WHERE product_name LIKE 'App%';
4. Misuse of Regular Expressions in REGEXP_LIKE
Issue
Inaccurate regular expressions may lead to unexpected matches or failures in `REGEXP_LIKE` queries.
Solution
Double-check the regular expression pattern and test it against sample data to verify its accuracy.
SQL Command-
SELECT * FROM customers WHERE REGEXP_LIKE(email, '^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$');
5. Performance Issues with Large Datasets
Issue
String comparison on large datasets may result in performance bottlenecks.
Solution
Optimize your queries by ensuring that relevant columns are indexed. Consider using efficient indexing strategies to speed up string comparison operations.
SQL Command-
CREATE INDEX idx_employee_name ON employees(employee_name);
6. Debugging Techniques
Issue
Encountering errors without clear information on what went wrong.
Solution
Use the `DBMS_OUTPUT.PUT_LINE` function to print debug information within your PL/SQL code. This aids in identifying where issues might be occurring.
SQL Command-
DECLARE
v_debug_info VARCHAR2(100);
BEGIN
-- Your code here
DBMS_OUTPUT.PUT_LINE('Debug Info: ' || v_debug_info);
END;
By addressing these common issues and employing the suggested solutions, you can enhance the accuracy and efficiency of your string comparison queries in Oracle SQL. Regular testing and a deep understanding of the specific challenges associated with string comparison will contribute to more robust and reliable database interactions.
Frequently Asked Questions
Can I compare strings based on linguistic sorting in Oracle SQL?
Yes, consider using STRING comparison functions like STRCMP, STRCOLL, and NLSSORT for linguistic sorting options.
What resources can I explore for further learning on string comparison in Oracle SQL?
Check recommended documentation, online communities, and books/articles listed in the “Resources and Further Reading” section of the article.
Conclusion
Comparing two strings in Oracle SQL involves selecting the right method based on your specific requirements. Whether you need a simple exact match, case-insensitive comparison, pattern matching, or more advanced linguistic sorting, Oracle SQL provides a range of tools to suit your needs.