Understanding Java isEmpty() vs isBlank()
In Java, isEmpty() and isBlank() are both methods used to check the emptiness of strings, but they have different behaviors and purposes. It’s important to understand the distinctions between isEmpty() and isBlank() to choose the appropriate method for your specific use case. In this article, we’ll explore the differences between isEmpty() and isBlank() in Java and guide when to use each.
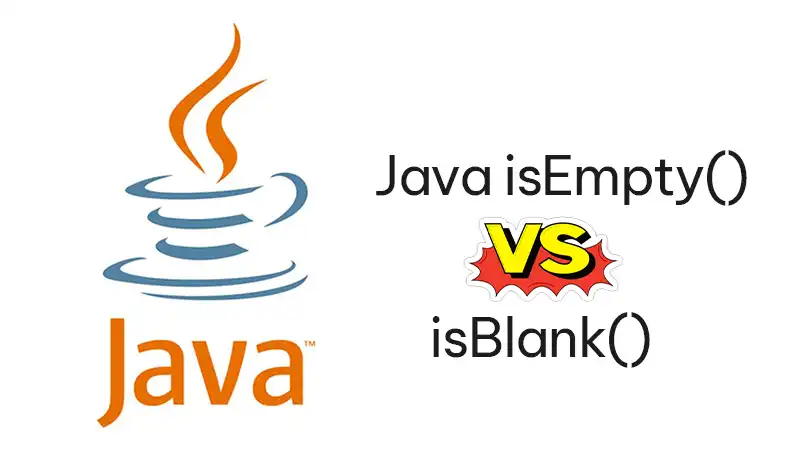
Overview of isEmpty()
The isEmpty() method is a part of the java.lang.String class in Java. It is used to check whether a string is empty, meaning it contains no characters. If the string has a length of zero, isEmpty() returns true; otherwise, it returns false.
Example
String str = "";
boolean empty = str.isEmpty(); // true
In this example, the isEmpty() method returns true because the string str has a length of zero.
Overview of isBlank()
The isBlank() method was introduced in Java 11 as part of the java.lang.String class. It is used to check whether a string is blank, meaning it contains only whitespace characters or has a length of zero. If the string is null or contains only whitespace characters, isBlank() returns true; otherwise, it returns false.
Example
String str = " ";
boolean blank = str.isBlank(); // true
In this example, the isBlank() method returns true because the string str contains only whitespace characters.
Differences and Use Cases
Behavior
isEmpty() checks whether a string has a length of zero, while isBlank() checks whether a string is null or contains only whitespace characters.
Use Cases
Use isEmpty() when you specifically want to check whether a string has no characters. Use isBlank() when you want to check whether a string is null or contains only whitespace characters, including spaces, tabs, and line breaks.
Frequently Asked Questions (FAQ)
What is the difference between a string being empty and being blank?
A string is considered empty if it has a length of zero, meaning it contains no characters. A string is considered blank if it is null or contains only whitespace characters.
Can I use isBlank() on older versions of Java?
No, isBlank() was introduced in Java 11, so it is not available in older versions of Java. If you need similar functionality in older versions, you can use libraries or custom methods to achieve the same result.
When should I use isEmpty() instead of isBlank()?
Use isEmpty() when you specifically want to check whether a string has no characters. For example, when validating user input to ensure a required field is not left blank.
Conclusion
In conclusion, isEmpty() and isBlank() are both useful methods for checking the emptiness of strings in Java, but they serve different purposes. isEmpty() checks whether a string has no characters, while isBlank() checks whether a string is null or contains only whitespace characters. Understanding the distinctions between isEmpty() and isBlank() can help you write more accurate and efficient Java code.