Webrootpath vs Contentrootpath | Comparison Guide
The two vital paths when building ASP.NET Core applications include the web root path (Webrootpath) and the content root path (Contentrootpath). WebRootPath is for public assets like CSS and images, accessible to browsers. ContentRootPath encompasses the entire source code and configurations, crucial for internal operations.
WebRootPath is within ContentRootPath, and they’re accessed through the IWebHostEnvironment interface in ASP.NET Core controllers. Customize paths using appsettings.json or environment variables. Razor syntax provides shortcuts for referencing assets in both paths.
Let’s contrast and leverage these fundamental directories.
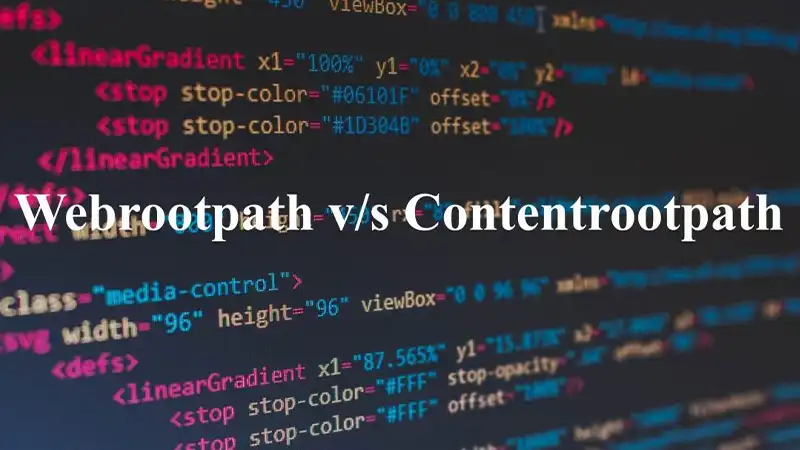
Webrootpath: Home of Public Assets
Think of Webrootpath as the public plaza in your application’s file structure. This specially designated path houses assets directly served to clients via the web server like HTML pages, CSS stylesheets, JavaScript files, images, and fonts.
Anything in Webrootpath becomes accessible by browser requests, composing the user interface and front-facing assets website visitors interact with.
Contentrootpath: Broader Internal Scope
In contrast, Contentrootpath encompasses a wider scope – your entire source code and any configuration files, database connections, runtime settings, or other code artifacts making up and driving your application.
Consider Contentrootpath as backstage to Webrootpath’s public-facing courtyard. The audience can’t directly access its contents, but it’s equally vital for the application machinery and workflows.
The Hierarchy: Webrootpath Within Contentrootpath
Importantly, Webrootpath lives under Contentrootpath in the directory structure. Remember:
Contentrootpath = Entire source code base Webrootpath = Public asset folder inside the source code base
This relationship means you can access Webrootpath files through Contentrootpath, but not vice-versa.
Typical Usage Scenarios
These distinctions dictate how each path gets used:
Webrootpath
- Storing front-end content served to clients like CSS, JS, images
- Referencing static assets in Razor views and MVC frameworks
Contentrootpath
- Housing-sensitive application code and configuration info
- Building absolute paths to non-public internal app resources
- Storing databases connections, API keys, and environmental variables
Retrieving the Paths
The IWebHostEnvironment interface in ASP.NET Core provides the Webrootpath and Contentrootpath properties for easy access:
public class MyController: Controller {
private readonly IWebHostEnvironment env;
public MyController(IWebHostEnvironment env) {
this.env = env;
}
public IActionResult Index() {
// Get paths
string webRoot = env.WebRootPath;
string contentRoot = env.ContentRootPath;
return View();
}
}
Injecting IWebHostEnvironment into your controllers lets you reference these paths anywhere.
Dynamically Configuring the Locations
While Webrootpath and Contentrootpath have conventional defaults, you can customize locations too:
Approaches:
- Specify non-standard paths in appsettings.json
- Set environment variables
- Leverage environments like Development vs. Production
For example:
{
"WebRootPath": "custom_public_assets",
"ContentRootPath": "custom_source_code"
}
This flexibility helps adapt file layouts across environments.
Static Assets Location: Webrootpath vs. Contentrootpath
Where should static files consumed internally by application code reside?
Rule of thumb:
- Browser-served assets: Webrootpath
- Code-only assets: Contentrootpath
This splits assets by public visibility.
Razor Syntax Shortcuts
Razor view engine provides shortcuts for referencing static assets:
<!-- Links CSS file in Webrootpath -->
<link href="~/styles.css" rel="stylesheet" type="text/css" />
<!-- Builds path to internal asset -->
@System.IO.Path.Combine(env.ContentRootPath, "assets", "logo.png")
The ~ points to Webrootpath while custom paths target Contentrootpath.
FAQs – Frequently Asked Questions and Answers
- Is it possible to store static files in Contentrootpath?
Answer: Yes, it’s possible, as long as they are consumed by internal application code and not directly served to the browser.
- Do both paths need to be explicitly defined?
Answer: No, both paths have default locations defined within ASP.NET Core. WebRootPath typically resides at the “wwwroot” folder, while ContentRootPath sits at the root of your project.
- Is it ever okay to put non-static files in WebRootPath?
Answer: Technically, yes, you can place any file within WebRootPath. However, it’s generally considered good practice to reserve this path solely for publicly accessible static files for security and clarity purposes.
To Conclude
Hopefully, this article has helped eliminate confusion differentiating ASP.NET Core’s Webrootpath and Contentrootpath. Leveraging their distinct identities unlocks smarter architectures, cleaner code, and mastery over the file system driving your applications. Happy coding.