Converting SQL Queries to LINQ | A Practical Guide
When transitioning from SQL to LINQ (Language-Integrated Query), developers often encounter a learning curve as the syntax and approach differ. However, understanding how to convert SQL queries to LINQ is crucial for those working with .NET languages like C# or VB.NET.
LINQ is a powerful feature in .NET languages that allows developers to query and manipulate data directly from within the code. While SQL is designed for querying databases, LINQ extends this capability to various data sources like arrays, collections, XML, and databases.
Let’s see how we can do it effortlessly.
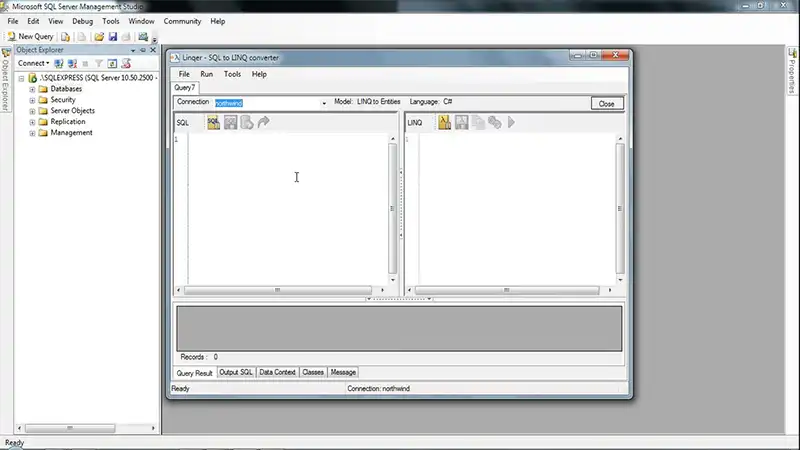
Basic SQL to LINQ Conversion
Let’s start with a simple SQL query and its LINQ equivalent:
SQL Query
SELECT FirstName, LastName FROM Users WHERE Age > 25;
LINQ Equivalent
var result = from user in dbContext.Users
where user.Age > 25
select new { user.FirstName, user.LastName };
In this example, we use LINQ’s from, where, and select clauses to mimic the SQL query structure. It’s important to note that LINQ encourages a more fluent and expressive syntax.
Filtering and Sorting
When dealing with more complex SQL queries involving filtering and sorting, the transition to LINQ involves adapting to its method-based syntax:
SQL Query
SELECT * FROM Products WHERE Category = 'Electronics' ORDER BY Price DESC;
LINQ Equivalent
var result = dbContext.Products
.Where(p => p.Category == "Electronics")
.OrderByDescending(p => p.Price);
In LINQ, we use the Where method for filtering and OrderByDescending for sorting. The lambda expressions provide concise conditions and projections.
Joining Tables
Handling joins is another essential aspect. Let’s convert a SQL join query to LINQ:
SQL Query
SELECT Orders.OrderID, Customers.CustomerName
FROM Orders
INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
LINQ Equivalent
var result = from order in dbContext.Orders
join customer in dbContext.Customers
on order.CustomerID equals customer.CustomerID
select new { order.OrderID, customer.CustomerName };
In LINQ, the join clause is used for joining tables based on specified conditions.
Aggregation Functions
When dealing with aggregations like SUM or COUNT, the translation involves using LINQ’s methods:
SQL Query
SELECT COUNT(*) FROM Products WHERE Price > 1000;
LINQ Equivalent
var count = dbContext.Products.Count(p => p.Price > 1000);
Here, the Count method in LINQ replaces the COUNT(*) SQL function.
Grouping Data
Grouping data is a common operation, and LINQ simplifies this process:
SQL Query
SELECT Category, AVG(Price) as AvgPrice
FROM Products
GROUP BY Category;
LINQ Equivalent
var result = from product in dbContext.Products
group product by product.Category into g
select new { Category = g.Key, AvgPrice = g.Average(p => p.Price) };
The group by clause in LINQ enables grouping based on a specified key.
Frequently Asked Questions
Can LINQ be used with non-relational data sources?
Yes, LINQ is not limited to relational databases. It can be used with various data sources, including arrays, collections, XML, and even custom data sources. The LINQ syntax may vary slightly depending on the type of data source, but the principles remain consistent.
How does error handling work in LINQ queries?
LINQ queries generally follow the same error handling principles as the rest of your .NET code. If there are issues with database connections, data retrieval, or any other part of the LINQ query, exceptions will be thrown. Developers should implement appropriate error-handling mechanisms, such as try-catch blocks, to handle exceptions gracefully.
Is there a performance difference between SQL and LINQ queries?
The performance of SQL and LINQ queries can vary based on factors such as the complexity of the query, the data source, and the underlying database. In some cases, well-optimized SQL queries might outperform equivalent LINQ queries.
However, LINQ provides a convenient and expressive way to work with data directly in code. Developers should be mindful of performance considerations and use tools like profiling to analyze and optimize code when needed.
Final Tips
While this guide covers the basics, it’s important to practice and explore LINQ’s capabilities further. LINQ provides a versatile and expressive way to query data within .NET applications, and mastering its syntax is beneficial for developers working with various data sources. As you become more familiar with LINQ, you’ll discover its flexibility and efficiency in handling diverse querying scenarios.