How to Save and Load RDD to Remote Hive Using Spark Scala? A Comprehensive Guide
Apache Spark, a powerful open-source distributed computing system, has gained widespread popularity for its speed and ease of use in processing large-scale data. When working with Spark in Scala, one common scenario is the need to save and load Resilient Distributed Datasets (RDDs) to and from a remote Apache Hive database.
This article aims to provide a comprehensive guide on how to accomplish this task using Spark Scala.
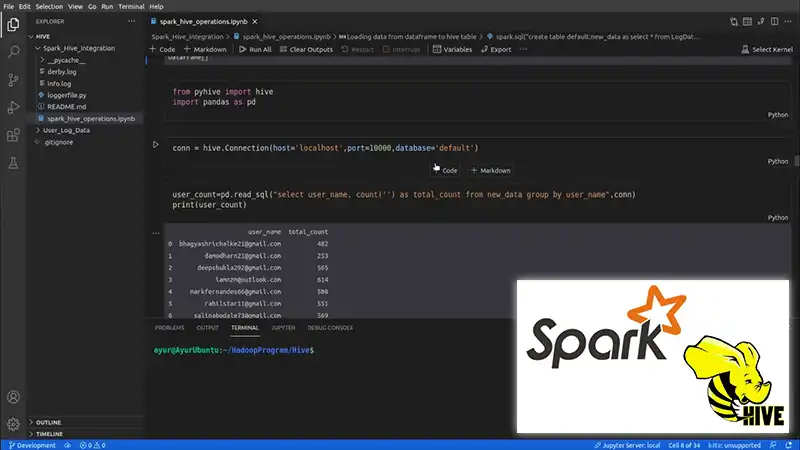
Prerequisites to Save and Load RDD to Remote Hive Using Spark Scala
Before diving into the process, ensure that you have the following prerequisites in place:
- Apache Spark is installed and configured on your machine.
- A running Apache Hive instance is accessible from your Spark environment.
- Setting up the Environment: Before we dive into the implementation, let’s make sure we have the necessary components in place. Ensure that you have Spark and Hive installed and properly configured on your system. Additionally, make sure you have a Hive database set up on a remote machine that can be accessed from your Spark cluster.
- Basic knowledge of Scala programming.
Saving RDD to Hive | 4 Steps
To save an RDD to a Hive table, follow these steps:
Step 1: Create a HiveContext:
Instantiate a HiveContext object, which provides access to the functionalities of Hive using Spark SQL.
val hiveContext = new HiveContext(sparkContext)
Step 2: Convert RDD to DataFrame:
RDDs can be converted to DataFrames using the toDF() method. This conversion is necessary because Hive works with DataFrames.
import hiveContext.implicits._val dataFrame = rdd.toDF()
Step 3: Register the DataFrame as a temporary table:
To interact with Hive, we need to register the DataFrame as a temporary table.
dataFrame.registerTempTable("temp_table")
Step 4: Insert data into the Hive table:
Next, we can use the HiveContext to insert the data from the temporary table into the desired Hive table.
hiveContext.sql("INSERT INTO TABLE hive_table SELECT * FROM temp_table")
Loading RDD from Hive | 5 Steps
Loading an RDD from a Hive table involves a similar process:
Step 1: Import Dependencies:
import org.apache.spark.sql.{Row, SparkSession}
Step 2: Create a Spark Session
val spark = SparkSession.builder .appName("LoadRDDFromHive") .config("spark.sql.warehouse.dir", warehouseLocation) .enableHiveSupport() .getOrCreate()
Step 3: Load Data from Hive Table
val df = spark.sql("SELECT * FROM your_hive_table_name")
Step 4: Convert DataFrame to RDD
val rdd: RDD[Row] = df.rdd
Step 5: Process or Display the RDD
Now that you have the RDD, you can perform further operations or display its contents as needed:
rdd.foreach(println)
Frequently Asked Questions
Can I save an RDD to Hive without using DataFrames in Spark Scala?
Yes, it’s possible. However, using DataFrames provides a more structured approach and is recommended for better integration with Spark’s capabilities.
What if my Hive instance is on a remote cluster?
Ensure that your Spark application has network connectivity to the remote Hive server. Adjust the configuration accordingly, and validate firewall settings if needed.
Can I load specific columns from a Hive table into an RDD?
Yes, when loading data from a Hive table into a DataFrame, you can select specific columns. The resulting DataFrame can then be converted to an RDD.
Are there performance considerations when saving/loading RDDs to/from Hive?
Yes, performance can be influenced by factors like data size, network latency, and cluster configuration. Optimize your Spark and Hive configurations for better efficiency.
How can I handle schema evolution when saving to Hive?
When saving to Hive, ensure that the RDD or DataFrame schema aligns with the Hive table schema. Handle any schema evolution by modifying your RDD or DataFrame accordingly before saving.
To Conclude
In this article, we explored how to save and load RDDs to a remote Hive database using Spark Scala. By following the steps outlined above, you can seamlessly integrate Spark and Hive to efficiently process and analyze large datasets. Leveraging the power of Spark Scala and Hive, you can unlock the true potential of your data analysis and processing workflows. Happy coding!