Resolving “Package oracle.jdbc.driver Does Not Exist” Error
Encountering errors during Java application development, particularly related to missing packages like “oracle.jdbc.driver,” can be a challenging obstacle to overcome. This error often signals a problem with locating the required Oracle JDBC driver package. In this article, we’ll explore the nuances of this error and provide actionable steps to resolve it effectively.
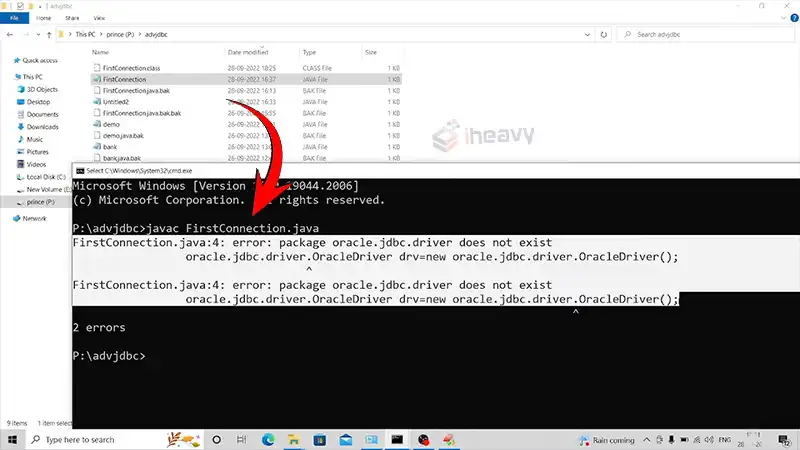
Deciphering the Error
When the message “Package oracle.jdbc.driver does not exist” appears, it’s a clear indication of trouble in accessing the necessary Oracle JDBC driver package. This package is vital for establishing connections to Oracle databases from Java applications.
Investigating Probable Causes
Understanding what might be causing this error is crucial:
Absent Oracle JDBC Driver
The absence of the Oracle JDBC driver JAR file within the classpath is the primary suspect when encountering this error.
Misconfigured Classpath
Incorrect classpath settings can lead to the compiler or runtime environment failing to locate the required JDBC driver package.
Dependency Management Snags
Issues with dependency declarations or version discrepancies, particularly when using tools like Maven or Gradle, can impede the resolution of the necessary JDBC driver.
Steps to Address the Issue
Resolving the “Package oracle.jdbc.driver does not exist” error involves taking the following steps:
Validate Oracle JDBC Driver Installation
Confirm that you have downloaded and installed the appropriate version of the Oracle JDBC driver for your Oracle database. The JDBC driver JAR file should be accessible on your system.
Review Classpath Configuration
Thoroughly check your Java project’s classpath configuration to ensure that it includes the directory or JAR file containing the Oracle JDBC driver. Verify these settings via command-line options, IDE configurations, or build tool setups.
Update Dependency Declarations
If you’re leveraging build automation tools such as Maven or Gradle, scrutinize your project configuration files (e.g., pom.xml for Maven) to ensure that the correct version of the Oracle JDBC driver is specified and that dependencies are resolved accurately.
Sample Java Code
import java.sql.*;
public class OracleJDBCExample {
public static void main(String[] args) {
try {
// Load Oracle JDBC driver
Class.forName("oracle.jdbc.driver.OracleDriver");
// Establish connection to Oracle database
Connection connection = DriverManager.getConnection(
"jdbc:oracle:thin:@//hostname:port/service_name", "username", "password");
// Perform database operations
// Close connection
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Frequently Asked Questions (FAQ)
Can I use alternative JDBC drivers for Oracle databases?
Yes, while the Oracle JDBC driver is recommended for optimal compatibility, there are third-party JDBC drivers available. However, thorough testing is necessary to ensure compatibility and performance.
How can I troubleshoot ClassNotFoundException related to the Oracle JDBC driver?
Make sure the JDBC driver JAR file is included in the classpath and that the correct package name is specified in the Class.forName() method.
Conclusion
The “Package oracle.jdbc.driver does not exist” error presents a hurdle in accessing the Oracle JDBC driver package required for Java applications to connect to Oracle databases. By addressing the root causes such as verifying driver installation, refining classpath configurations, and rectifying dependency management issues, developers can overcome this error and establish successful connections to Oracle databases.