How to Use Prepared Statements Within MySQL Stored Procedures? | Explained
MySQL stored procedures are powerful database objects that allow developers to encapsulate a sequence of SQL statements into a reusable and efficient block of code. When it comes to enhancing the security and performance of your MySQL stored procedures, leveraging prepared statements is key.
Prepared statements help prevent SQL injection attacks and optimize query execution. In this article, we’ll explore how to harness the benefits of prepared statements within MySQL stored procedures.
What are Prepared Statements?
A prepared statement is a precompiled SQL statement that can be executed multiple times with different parameters. Unlike regular SQL queries, prepared statements separate SQL logic from data, reducing the risk of SQL injection. This separation also improves performance by allowing the database engine to cache and reuse the execution plan for the statement.
Benefits of Using Prepared Statements in Stored Procedures
Using prepared statements is particularly advantageous as it promotes code reusability and maintenance.
Security: Prepared statements protect against SQL injection attacks, ensuring that user input does not interfere with the structure of the SQL query.
Performance: The use of prepared statements allows MySQL to optimize query execution by reusing the execution plan for multiple executions, resulting in improved performance.
Readability and Maintainability: Separating SQL logic from data enhances code readability and maintainability, making it easier for developers to understand and update stored procedures.
Steps to Use Prepared Statements Within MySQL Stored Procedures
Here’s a basic guide on how to use prepared statements in MySQL stored procedures:
1. Create a Stored Procedure
Start by creating a stored procedure using the CREATE PROCEDURE statement. Here’s a simple example:
- DELIMITER //
- CREATE PROCEDURE GetUserInfo(IN userId INT)
- BEGIN
- — Your SQL statements go here
- END //
- DELIMITER ;
In this example, the stored procedure named GetUserInfo takes an input parameter userId of type INT.
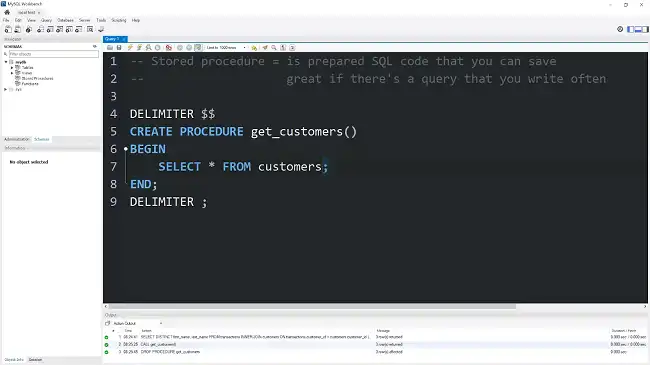
2. Declare Variables
Declare variables to hold the values of the parameters and any other temporary variables you might need within the stored procedure. For example:
- DELIMITER //
- CREATE PROCEDURE GetUserInfo(IN userId INT)
- BEGIN
- DECLARE userName VARCHAR(255);
- — Your SQL statements go here
- END //
- DELIMITER ;
3. Use PREPARE Statement
Inside the stored procedure, use the PREPARE statement to create a prepared statement. The syntax is as follows:
- PREPARE statement_name FROM ‘your_sql_query’;
For example:
- DELIMITER //
- CREATE PROCEDURE GetUserInfo(IN userId INT)
- BEGIN
- DECLARE userName VARCHAR(255);
- — Create a prepared statement
- PREPARE stmt FROM ‘SELECT username FROM users WHERE id = ?’;
- — Your SQL statements go here
- — Deallocate the prepared statement
- DEALLOCATE PREPARE stmt;
- END //
- DELIMITER ;
Note the use of the ‘?’ as a placeholder for the parameter.
4. Bind Parameters
Use the SET statement to bind values to the parameters in the prepared statement. In this example, bind the userId parameter:
- SET @user_id = userId;
Then, execute the prepared statement using the EXECUTE statement:
- EXECUTE stmt USING @user_id;
5. Handle Result
Use the FETCH statement to retrieve the result of the prepared statement into variables:
- FETCH stmt INTO userName;
You can then use the ‘userName’ variable in your stored procedure as needed.
6. Deallocate Prepared Statement
Finally, use the DEALLOCATE statement to free up resources associated with the prepared statement:
- DEALLOCATE PREPARE stmt;
Here’s the complete example:
- DELIMITER //
- CREATE PROCEDURE GetUserInfo(IN userId INT)
- BEGIN
- DECLARE userName VARCHAR(255);
- — Create a prepared statement
- PREPARE stmt FROM ‘SELECT username FROM users WHERE id = ?’;
- — Bind parameter
- SET @user_id = userId;
- — Execute the prepared statement
- EXECUTE stmt USING @user_id;
- — Fetch result into variable
- FETCH stmt INTO userName;
- — Use the result as needed
- — Deallocate the prepared statement
- DEALLOCATE PREPARE stmt;
- END //
- DELIMITER ;
Remember to adapt the SQL statements and parameter names to fit your specific use case.
Frequently Asked Questions
Can prepared statements be used in all types of MySQL stored procedures?
Yes, prepared statements can be utilized in all types of MySQL stored procedures, including those with conditional logic and loops.
Can we call stored procedures inside stored procedures in MySQL?
Invoking one stored procedure from another is straightforward. This can be achieved using the CALL statement, similar to how you would do it through the MySQL command-line client.
When not to use prepared statements?
If you’re certain that the string originates from your application and is not susceptible to manipulation by a user, then there’s no necessity for prepared statements since there is no potential for injection.
Conclusion
Using prepared statements within MySQL stored procedures is a best practice that enhances both security and performance. By separating SQL logic from user input, you not only safeguard your database but also optimize query execution. Experiment with these techniques in your projects, and don’t hesitate to reach out with any questions or feedback. Thank you for taking the time to read this article.