How to Implement Authorization Code Flow With OWIN | Explained
Implementing the Authorization Code Flow with OWIN (Open Web Interface for . NET) is a crucial step in ensuring secure authentication and authorization within web applications. This flow is widely used to grant permissions and access tokens to users and applications securely.
By following a few key steps, developers can integrate this flow seamlessly into their .NET projects using OWIN.
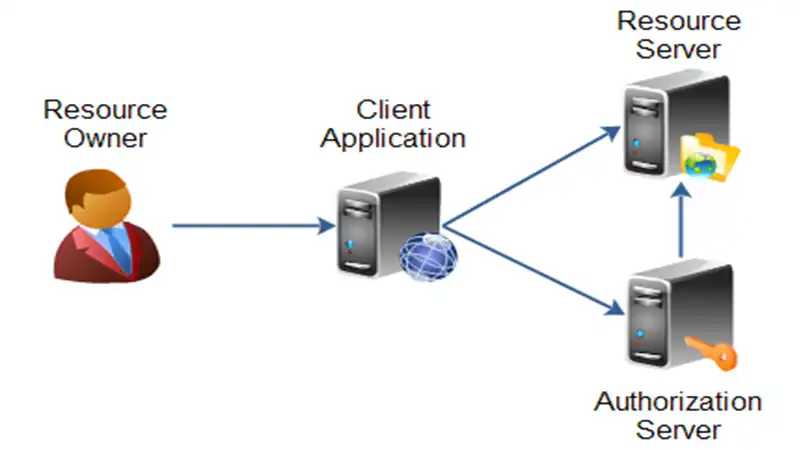
Exploring Authorization Code Flow
The Authorization Code Flow is an OAuth 2.0 authentication method designed for web applications that need access to secure resources. It involves multiple steps:
1. User Authorization: The user requests access to a protected resource.
2. Authorization Grant: The application requests authorization from the user via a redirection to the authorization server.
3. User Authentication: The user provides credentials and consents to grant access.
4. Authorization Code: Upon successful authentication, the authorization server issues an authorization code to the application.
5. Token Exchange: The application exchanges this code for an access token.
6. Accessing Resources: The application uses the access token to access protected resources.
Implementing Authorization Code Flow with OWIN
To implement Authorization Code Flow using OWIN in your .NET application, follow these steps:
Step 1: Set Up the OAuth Middleware
Use the OAuth middleware provided by OWIN to configure authentication with the authorization server. This middleware handles the interactions between your application and the authorization server.
Step 2: Configure Client Credentials
Provide the necessary client credentials (Client ID and Client Secret) obtained during your application registration with the authorization server. These credentials uniquely identify your application to the authorization server.
Step 3: Define Callback URLs
Specify the callback URLs where the authorization server will redirect users after they authenticate and authorize your application. These URLs are used to retrieve the authorization code.
Step 4: Handle Authentication Callbacks
Implement logic to handle the callback from the authorization server. Extract the authorization code from the callback and exchange it for an access token by making a POST request to the authorization server’s token endpoint.
Step 5: Securely Store Tokens
Once you receive the access token, securely store it. Use this token to access protected resources on behalf of the user.
Sample Code (Using OWIN and ASP.NET)
Here’s an example of how you might configure OWIN middleware for Authorization Code Flow:
app.UseOAuthAuthorizationServer(new OAuthAuthorizationServerOptions
{
TokenEndpointPath = new PathString(“/token”),
AuthorizeEndpointPath = new PathString(“/authorize”),
AccessTokenExpireTimeSpan = TimeSpan.FromMinutes(30),
Provider = new CustomAuthorizationServerProvider(),
// Other configurations
});
app.UseOAuthBearerAuthentication(new OAuthBearerAuthenticationOptions());
Frequently Asked Questions
Can Authorization Code Flow be used for mobile or single-page applications (SPAs)?
While Authorization Code Flow is primarily designed for server-side applications due to its ability to protect sensitive information like client secrets, techniques like PKCE (Proof Key for Code Exchange) can be used to adapt this flow for SPAs or mobile apps securely.
Can multiple applications share the same authorization server in Authorization Code Flow?
Yes, multiple applications can use the same authorization server to authenticate users and obtain access tokens. Each application is typically identified by its unique client credentials (Client ID and Secret).
Is OWIN the only way to implement Authorization Code Flow in .NET applications?
No, OWIN is a popular choice due to its flexibility. Still, there are other libraries and frameworks available in the .NET ecosystem that can also facilitate Authorization Code Flow implementation, such as IdentityServer or ASP.NET Core Identity.
Conclusion
Implementing the Authorization Code Flow with OWIN in .NET applications ensures secure resource access while maintaining user privacy and security. By understanding the flow and following the steps outlined, developers can seamlessly integrate this authentication method into their applications, providing a robust and secure user experience.