Identify With Odd and Even Rows in T-SQL Tables: A Practical Guide
Working with SQL often involves addressing specific scenarios, and one common requirement is dealing with odd and even rows in a table. This becomes particularly crucial when striving to organize and analyze data systematically.
In this article, we’ll go through two straightforward approaches to tackle this task effectively. The first method employs a temporary table, providing a simple way to discern between odd and even rows. However, the second method involves leveraging a Common Table Expression (CTE) and the ROW_NUMBER() function.
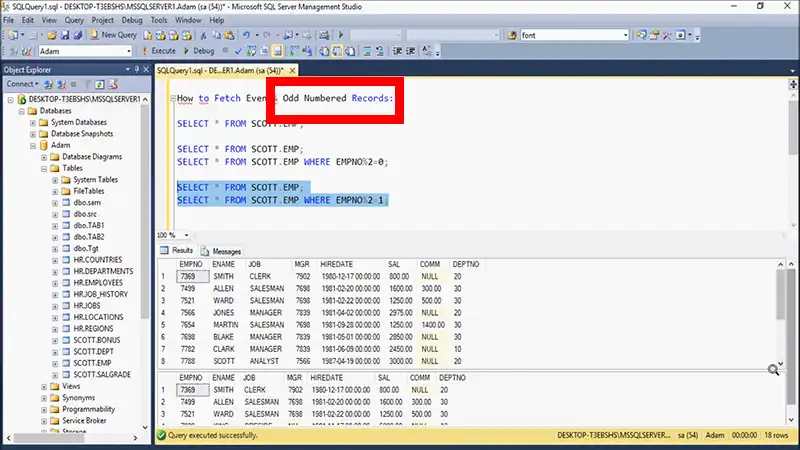
How to Identify With Odd and Even Rows
Below are the two methods we’ve been talking about so far. You can choose either or experiment with both to see what sticks.
Approach 1: Using a Temporary Table
The first method employs a temporary table, providing a simple way to discern between odd and even rows.
1. Create a Temporary Table
Start by crafting a new table with an “id” column that counts up from 1 for each row, along with a column to hold your data (let’s call it “order_num”). Here’s the demo.
CREATE TABLE #tbl_temp (
id INT IDENTITY(1, 1) PRIMARY KEY,
order_num INT
);
The above statement creates a temporary table named #tbl_temp with two columns: id, which is an automatically incremented primary key, and order_num, which is a column where you can store your data. You can use it for various purposes, such as the identification of odd and even rows, as demonstrated in the provided SQL examples. Keep in mind that the #tbl_temp table is temporary and will only exist for the duration of the session or query execution.
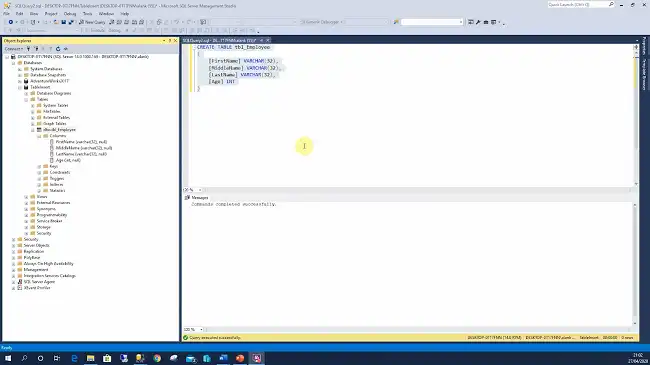
2. Populate the Temporary Table
Transfer the data from your original table to the new temporary table using the following query.
INSERT INTO #tbl_temp (order_num)
SELECT order_num
FROM original_table;
Here, we are inserting values from the order_num column of the original_table into the order_num column of the temporary table #tbl_temp. It essentially transfers data from one table to another, allowing us to manipulate or analyze the data in the temporary table without affecting the original data in original_table.
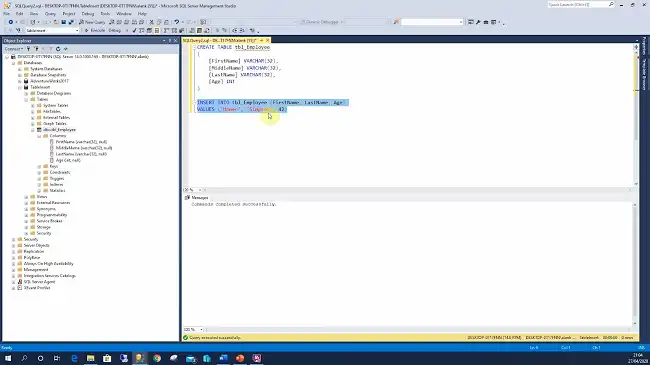
3. Identify Odd and Even Rows
Now, you can easily identify odd and even rows based on the “id” column. Here’s how.
— Odd Rows
SELECT order_num
FROM #tbl_temp
WHERE id % 2 = 1;
-- Even Rows
SELECT order_num
FROM #tbl_temp
WHERE id % 2 = 0;
Approach 2: Using a CTE and ROW_NUMBER() Function
The second method involves leveraging a Common Table Expression (CTE) and the ROW_NUMBER() function.
1. Create a CTE with Row Numbers
Generate a CTE that assigns a row number to each row in your original table.
;WITH cte AS
(
SELECT order_num, ROW_NUMBER() OVER (ORDER BY order_num) AS row_num
FROM original_table
)
This SQL statement creates a Common Table Expression (CTE) named `cte` that includes the `order_num` column and assigns a row number (`row_num`) based on the order of values in the `order_num` column from the `original_table`.
2. Identify Odd Rows
Utilize the CTE to easily identify odd rows.
SELECT order_num
FROM cte
WHERE row_num % 2 = 1;
What this statement does is it selects values from the `order_num` column of the Common Table Expression (CTE) named `cte`, filtering for rows where the assigned row number (`row_num`) is odd.
Frequently Asked Questions
Can I apply odd and even row identification in SQL queries for pagination purposes?
Yes, it is a common practice. Knowing whether a row is odd or even can assist in creating dynamic pagination displays in user interfaces.
How do I show odd and even rows in SQL?
Retrieve all records from the table where the values in the specified column are even using the syntax: `SELECT * FROM table_name WHERE column_name % 2 = 0;`. To identify rows with odd values in the target column, use: `SELECT * FROM table_name WHERE column_name % 2 <> 0;`. It’s important to note that SQL Server lacks a MOD function.
Conclusion
In conclusion, this guide introduces two efficient methods to work with odd and even rows in a T-SQL table. Whether you choose the temporary table approach or opt for the CTE and ROW_NUMBER() method depends on the specific task at hand. These techniques offer flexibility and can be tailored to your unique requirements.
Have another method in mind? Share it here! Your insights are valuable, and we may even feature them in a follow-up blog post. Thanks for reading!