Is It Possible to Use Event Handlers in ASP Net Web API Controllers?
Event handling is a crucial aspect of any robust application. But when it comes to ASP.NET Web API, confusion often arises regarding the use of event handlers within controllers.
Yes, it is possible to use event handlers in ASP.NET Web API controllers. This can be achieved through techniques like Dependency Injection (DI), static classes, or message queues, each with its own trade-offs in terms of simplicity, testability, and decoupling. The choice depends on the specific requirements and priorities of the application’s architecture.
This comprehensive guide will dive deep into whether ASP.NET Web API controllers can leverage event handlers.
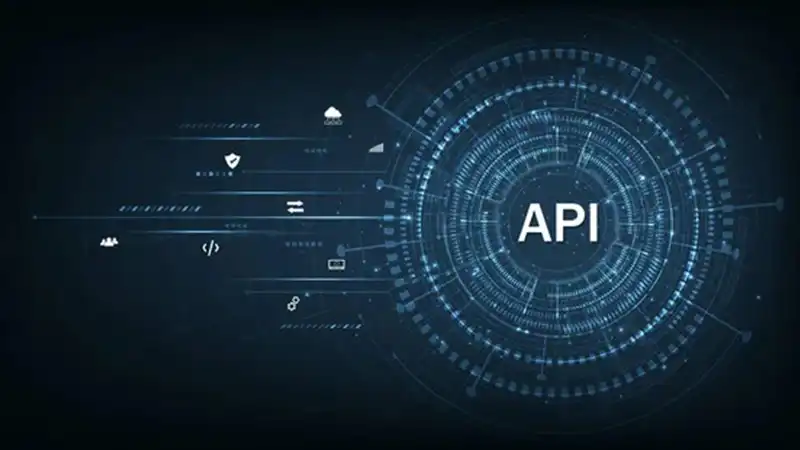
The Role of Controllers and Events
First, a quick refresher. ASP.NET Web API controllers handle incoming HTTP requests and return responses. Events signal specific occurrences, triggering associated actions. Event handlers encapsulate that response logic.
The Decoupling Dilemma
Typically, event handlers reside in separate classes, decoupled from controllers. However, controllers have brief lifecycles, created per request. Registering handlers in them leads to tight coupling and impaired execution.
Breaking Conventional Chains
Despite traditional constraints, ingenious techniques exist for using handlers in Web API controllers:
- Dependency Injection: Inject a handler instance into controllers via Dependency Injection (DI). This promotes loose coupling and testability.
- Static Classes: House handlers in static classes, ensuring availability throughout the application lifetime. However, testability suffers.
- Message Queues: Use queues to unlink events and handlers completely. Controllers publish events to the queue. Subscribers asynchronously process them.
Evaluating the Trade-Offs
Each approach carries unique trade-offs:
- DI: Easy to implement but demands solid DI mastery.
- Static Classes: Simple but testing and thread-safety prove challenging.
- Queues: Decoupled and robust but increases complexity.
Choosing the right solution depends on your architecture and priorities.
Hands-on Example: DI Approach
Let’s implement the DI approach, injecting an event handler into the controller:
// Interface for the handler
public interface IOrderPlacedEventHandler
{
void Handle(object sender, OrderPlacedEventArgs args);
}
// Event args class
public class OrderPlacedEventArgs : EventArgs
{
public Order Order { get; set; }
}
// Controller with injected handler
public class OrdersController : ApiController
{
private readonly IOrderPlacedEventHandler _handler;
public OrdersController(IOrderPlacedEventHandler handler)
{
_handler = handler;
}
// Trigger event on order creation
public async Task<IHttpActionResult> PostOrder(Order order)
{
// Save order
_handler.Handle(this, new OrderPlacedEventArgs { Order = order});
return Ok();
}
}
// Concrete implementation of handler
public class SendOrderPlacedNotificationHandler: IOrderPlacedEventHandler
{
public void Handle(object sender, OrderPlacedEventArgs args)
{
// Logic to send notification
}
}
This separates the event handling concern from the controller’s logic.
Architectural Considerations
Carefully evaluate needs before introducing eventing complexity:
- Multi-purpose: If reusing handler logic across contexts, eventing shines
- Extensibility: Events simplify adding future handler logic
- Request/Response: If controller action initiates and finishes a workflow, less useful
Testing Event Handlers
Unit test handlers just like any component:
// Mock dependencies
var mockHandler = new Mock<IOrderPlacedEventHandler>();
// Set expectations
mockHandler.Setup(h =>
h.Handle(It.IsAny<object>(), It.IsAny<OrderPlacedEventArgs>())
);
// Trigger event
ordersController.PostOrder(new Order());
// Assert if expectation met
mockHandler.VerifyAll();
FAQs – Frequently Asked Questions and Answers
- Is it possible to use static event handlers in Web API Controllers?
Answer: Yes, you can utilize static event handler classes that remain memory-resident. But carefully consider thread safety, testability, and lifespan management.
- Is it a good practice to subscribe to events in Web API controllers?
Answer: Avoid subscribing to events directly in Web API controllers due to their limited lifecycles. Consider injecting handlers via DI or using static classes instead.
- How to test event handlers used in Web API controllers?
Answer: Use a mocking framework to set expectations and assertions around the handler methods. Trigger controller actions to fire events and then verify expected handler behavior.
To Conclude
In closing, implementing event handlers in Web API controllers is achievable through various creative techniques. Each approach carries unique trade-offs to evaluate. Use case, architecture, and priorities should guide your technology choice. Mastering event-driven programming unlocks game-changing possibilities for organizing logic in enterprise applications.