How to Eliminate Columns With Empty Names in CSV File
Dealing with CSV files is a routine task for many, but it’s not uncommon to encounter challenges like columns with empty names. These seemingly blank columns can get in the way of your data processing workflow, especially when you’re trying to clean the data.
In this guide, we’ll explore the steps to eliminate columns with empty names in a CSV file, ensuring a smoother and more efficient data manipulation process. So, without further delay, let’s dive right in.
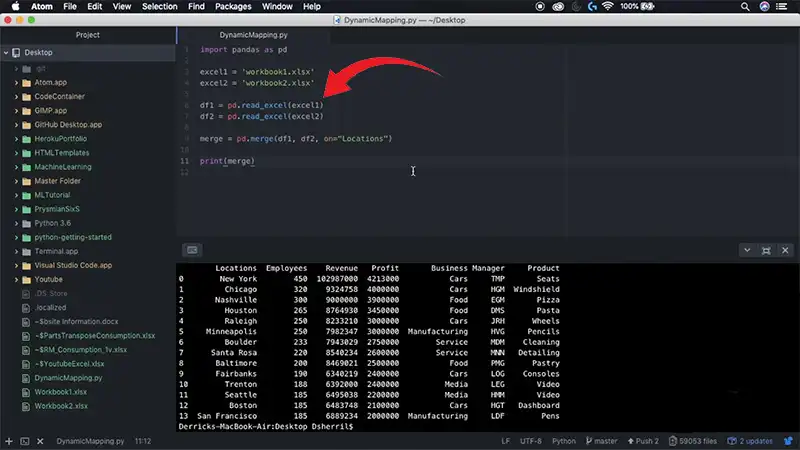
Eliminating Columns in Different Ways With Empty Names in CSV File
In this section, we explore various methods to effectively eliminate columns with empty names in a CSV file. We’ll delve into four distinct approaches, each catering to different preferences and toolsets.
Eliminate Columns With Empty Names Using Excel
In Excel, you can eliminate columns with empty names by manually selecting and deleting them. However, if your CSV file has a large number of columns and you want to automate this process, you might consider using a VBA macro like the following.
Sub DeleteColumnsWithEmptyNames()
Dim ws As Worksheet
Dim lastColumn As Long
Dim i As Long
‘ Set the worksheet where you want to perform the operation
Set ws = ThisWorkbook.Sheets(“Sheet1”) ‘ Change “Sheet1” to your sheet name
‘ Find the last column with data in the worksheet
lastColumn = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column
‘ Loop through columns from right to left and delete columns with empty names
For i = lastColumn To 1 Step -1
If ws.Cells(1, i).Value = “” Then
ws.Columns(i).Delete
End If
Next i
End Sub
Here’s how you can use this code:
- Press Alt + F11 to open the VBA editor in Excel.
- Insert a new module by right-clicking on any item in the Project Explorer, selecting Insert, and then choosing Module.
- Copy and paste the code into the module.
- Close the VBA editor.
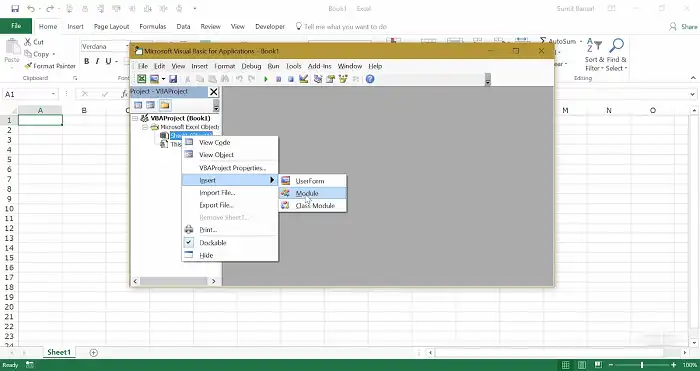
Now you can run this macro by pressing Alt + F8, selecting DeleteColumnsWithEmptyNames, and clicking Run.
Note: Always test macros on a copy of your data to avoid accidental loss of important information.
Eliminate Columns With Empty Names Using Python and pandas
Assuming you have a CSV file named “your_file.csv,” here’s a step-by-step guide to eliminate columns with empty names using python.
import pandas as pd
# Load the CSV file into a DataFrame
df = pd.read_csv(‘your_file.csv’)
# Drop columns with empty names
df = df.dropna(axis=1, how=’all’)
# Save the modified DataFrame to a new CSV file
df.to_csv(‘new_file.csv’, index=False)
How it works:
pd.read_csv(‘your_file.csv’): Reads the CSV file into a pandas DataFrame.
df.dropna(axis=1, how=’all’): Drops columns with all NaN (empty) values along the column axis.
df.to_csv(‘new_file.csv’, index=False): Saves the modified DataFrame to a new CSV file without including the index column.
Eliminate Columns With Empty Names Using awk (Unix/Linux command-line tool)
You can also eliminate columns with empty names by running the following command line. Make sure to keep a backup of your CSV file before executing the code.
awk -F, ‘NR==1 {for(i=1;i<=NF;i++) if($i!=””) c[++j]=$i} {for(i=1;i<=j;i++) printf “%s%s”, $(c[i]), (i<j ? OFS : ORS)}’ your_file.csv > new_file.csv
How it works:
NR==1 {for(i=1;i<=NF;i++) if($i!=””) c[++j]=$i}: For the first row, it creates an array c containing the column indices with non-empty names.
{for(i=1;i<=j;i++) printf “%s%s”, $(c[i]), (i<j ? OFS : ORS)}: For each row, it prints the selected columns separated by the output field separator (OFS), and at the end of each line, it uses the output record separator (ORS).
your_file.csv > new_file.csv: Redirects the output to a new file.
Eliminate Columns With Empty Names in Azure Data Factory
Azure Data Factory (ADF) is a cloud-based data integration service that allows you to create data-driven workflows for orchestrating and automating data movement and data transformation. If you want to eliminate columns with empty names in a CSV file using Azure Data Factory, you can achieve this using the following steps:
1. Use a Copy Data activity:
Start by creating a pipeline in Azure Data Factory.
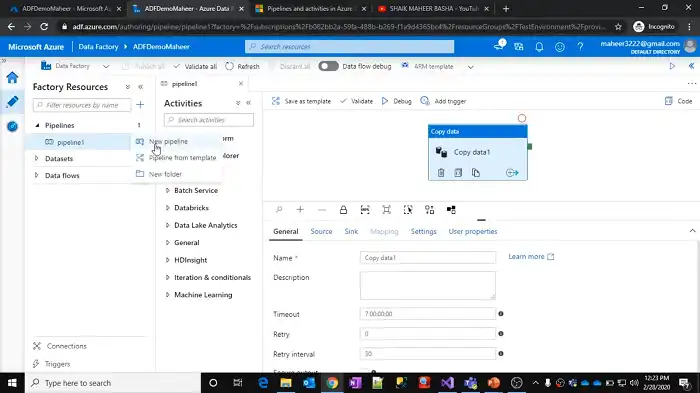
Add a Copy Data activity to your pipeline.
2. Configure Source Dataset:
Configure the source dataset to point to your CSV file.
Ensure that the dataset settings correctly reflect the structure of your CSV file.
3. Configure Sink Dataset:
Configure the sink dataset, which is where the modified data will be written.
The sink dataset can be a new CSV file or the same CSV file with overwrite settings.
4. Use Mapping in Copy Data Activity:
In the Copy Data activity, use the Mapping settings to define the column mappings between the source and sink datasets.
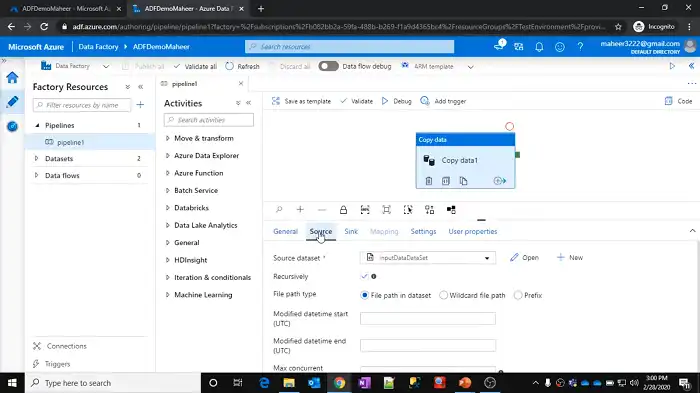
Open the Mapping settings, and you should see a list of columns from the source and sink datasets.
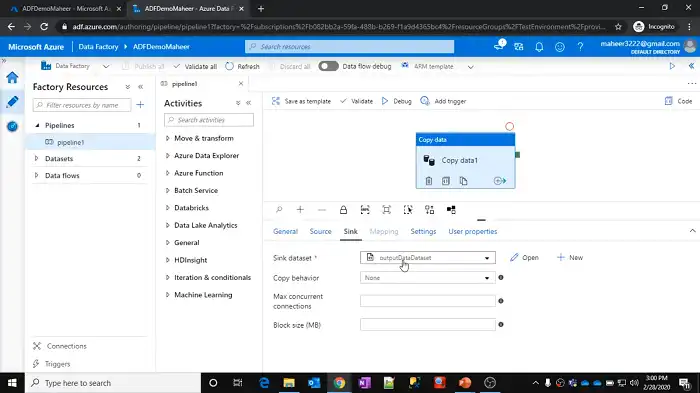
5. Filter Columns:
Add a column transformation to filter out columns with empty names.
You can use the following expression in the mapping to achieve this:
{
“type”: “TabularTranslator”,
“mappings”: [
{
“source”: {
“name”: “ColumnName”
},
“sink”: {
“name”: “ColumnName”,
“applyto”: “copyIfNotNull”
}
},
// Add similar mappings for other columns
]
}
This mapping expression ensures that only columns with non-empty names are copied to the sink.
6. Run the Pipeline:
Save your changes and run the pipeline. The Copy Data activity will now use the specified mapping to eliminate columns with empty names during the data copy process.
Remember to adjust the column names and settings according to your specific CSV file structure. The key here is to use the Mapping settings in the Copy Data activity to filter out columns based on your criteria.
FAQs Time
How do I find blank columns in Excel?
To find blank columns in Excel, you can use the “Go To Special” feature. Press `Ctrl + G` to open the “Go To” dialog, choose “Special,” select “Blanks,” and click “OK” to highlight all blank cells. If the entire column is blank, the entire column will be selected.
Will removing empty header columns affect my original data?
No, the steps provided create a new CSV file with the problematic columns removed, leaving your original data intact.
What if I have multiple CSV files with the same issue?
You can iterate through multiple files using a loop or a function, applying the same steps to each file.
Conclusion
Handling CSV files that have columns with empty names might seem daunting, but with the right tools and a bit of coding knowledge, it becomes a manageable task. By following the steps outlined in this guide, you can streamline your data processing workflow and save valuable time. If you have any questions or feedback, feel free to reach out. Happy data cleaning!