How To Fetch Data From Database In WordPress Plugin | 3 Fascinating Ways
WordPress is a versatile platform known for its flexibility and extensibility, and one of the common tasks plugin developers often need to perform is fetching data from the database. In this article, we’ll explore three fascinating methods to accomplish this task, each with its unique advantages.
We’ll delve into the use of WP_Query, get_posts(), and the $wpdb class, offering you a comprehensive guide to make informed decisions when working with database-related operations in WordPress plugins
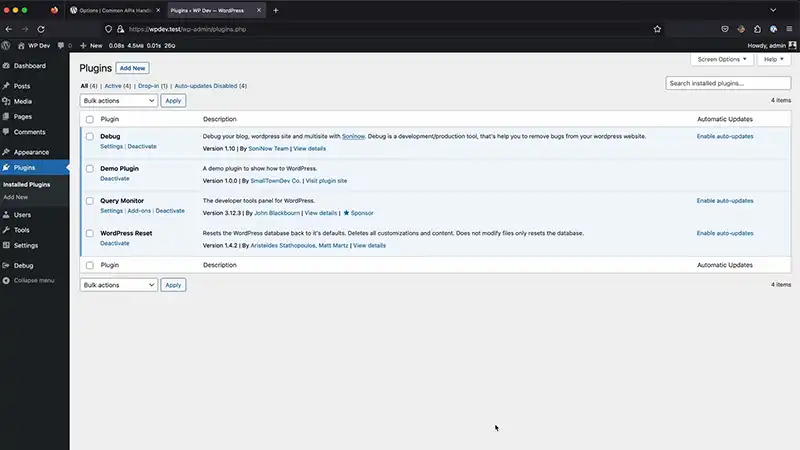
Fetch Data From Database In WordPress Plugin
In WordPress, you’ve got three main ways to get info from the database. These methods come with different levels of complexity and flexibility, so you can pick the one that works best for your unique needs.
- WP_Query: This is the primary query method used in WordPress to retrieve posts and other content from the database. It offers a wide range of parameters and is highly customizable.
- get_posts(): This is a simpler alternative to WP_Query and is used specifically to retrieve posts. It provides a simpler and more concise way to fetch post data.
- $wpdb (WordPress Database Class): For more advanced database operations, you can use the global $wpdb object. It allows you to write custom SQL queries and interact with the database directly.
1. WP_Query
To get data from your WordPress database, start with queries. These are like easy commands for fetching information.
You can use queries to pull all your website’s content from the database. They also come with a loop that formats your data to match your website’s design.
WP-Query() is a helpful tool that can do many things. Here’s a simple code example to get data from your WordPress database on a page:
<?php
// The Query
$the_query = new WP_Query( $args );
// The Loop
if ( $the_query->have_posts() ) {
echo '<ul>';
while ( $the_query->have_posts() ) {
$the_query->the_post();
echo '<li>' . get_the_title() . '</li>';
}
echo '</ul>';
} else {
// no posts found
}
/* Restore original Post Data */
wp_reset_postdata();
?>
2. get_posts():
In this instance, we create an array called `$args` to set specific preferences for our query. These preferences include details like the type of posts we want, the quantity of posts to retrieve, and how they should be ordered and sorted. We then employ the `get_posts($args)` function to gather the posts based on these preferences.
Within the loop, we utilise functions such as `get_the_title()` and `get_the_excerpt()` to exhibit the post information. To prevent any potential conflicts with other aspects of your WordPress site, we wrap up by resetting the post data using `wp_reset_postdata()`.
Here’s a simple code example to get data from your WordPress database on a page:
<?php
// Define your arguments
$args = array(
'post_type' => 'post', // Specify the post type (e.g., 'post', 'page', 'custom_post_type')
'posts_per_page' => 5, // Number of posts to retrieve
'orderby' => 'date', // Order by date
'order' => 'DESC' // Sort in descending order (newest first)
);
// Get the posts based on the arguments
$posts = get_posts($args);
// Check if there are posts
if ($posts) {
foreach ($posts as $post) {
setup_postdata($post); // Set up post data for use in the loop
// Output post information
echo '<h2>' . get_the_title() . '</h2>';
echo '<p>' . get_the_excerpt() . '</p>';
}
// Reset post data
wp_reset_postdata();
} else {
echo 'No posts found.';
}
?>
3. $wpdb (WordPress Database Class)
Here we make the $wpdb tool accessible in our code so we can use it effectively. Then, we create a special request to the database, asking for IDs and post titles, but only for posts that are of type ‘post’ and are currently published.
Next, we send this request to the database using $wpdb->get_results(), which brings back the results in a neat list. We go through this list and show the ID and title for each post.
If, by any chance, we don’t find any posts that match our request, we tell the user that there’s nothing to show.
Always remember to be careful when working with custom database requests like this. Mistakes in these requests can be harmful to your website’s database. To keep things secure and reliable, it’s important to make sure your data is clean and your requests are safe.
Here’s a simple code example to get data from your WordPress database on a page:
global $wpdb;
// Define your custom SQL query
$query = "SELECT ID, post_title FROM $wpdb->posts WHERE post_type = 'post' AND post_status = 'publish'";
// Run the query
$results = $wpdb->get_results($query);
// Check if there are results
if ($results) {
foreach ($results as $post) {
echo 'Post ID: ' . $post->ID . ' - Post Title: ' . $post->post_title . '<br>';
}
} else {
echo 'No posts found.';
}
Frequently Asked Questions
- What is WP_Query, and how does it differ from get_posts()?
WP_Query is a powerful WordPress class used to query and retrieve posts from the database. It offers extensive customization options and is ideal for creating complex queries with specific criteria.
On the other hand, get_posts() is a simpler function that returns an array of posts based on a set of parameters. WP_Query is more flexible and versatile, while get_posts() is easier to use for basic queries.
- When should I use the $wpdb class in my WordPress plugin?
The $wpdb class is best suited for executing custom SQL queries in WordPress plugins. It provides direct access to the WordPress database and is especially useful when you need to perform complex operations or retrieve data from non-standard database tables.
However, it should be used with caution, as direct database manipulation can be risky if not handled properly.
- Are there any performance considerations when fetching data from the database in a WordPress plugin?
Yes, performance is a crucial aspect to consider when working with database queries in WordPress plugins. Using efficient queries, caching mechanisms, and limiting the number of database calls can help improve your plugin’s performance.
Additionally, proper indexing and optimization of your database tables can also play a significant role in ensuring your plugin runs smoothly and doesn’t cause undue strain on your WordPress site
Conclusion:
To sum it up, in WordPress, you have three ways to get data from the database, each with its own complexity and customization options. WP_Query is the flexible choice for most needs, get_posts() is a simpler way to fetch posts, and $wpdb lets you do advanced stuff with custom SQL queries. You get to pick what suits your needs best for managing and showing content.