How to Add Leading Zeros in SQL | 4 Methods and More
In the vast universe of SQL, adding leading zeros might seem like an insignificant constellation. However, these seemingly unassuming zeros play a crucial role in data formatting and organization. It is particularly relevant when dealing with codes or identifiers where we must maintain a consistent format.
Understanding how to add leading zeros in SQL can make all the difference in how you present and process your data. Let’s embark on this journey to grasp why leading zeroes are important and how to add them in SQL.
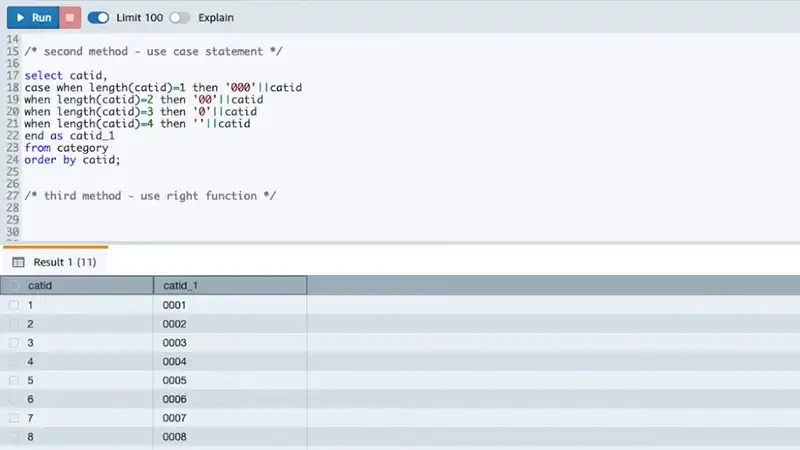
Why Add Leading Zeros in SQL?
Before delving into the intricacies of the how, let’s ponder the why. Adding leading zeros in SQL is not merely a matter of aesthetics; it is a pragmatic necessity. The necessity arises from the need to ensure consistent data formatting, especially when dealing with alphanumeric identifiers.
Various systems and applications rely on uniform data formatting for accurate processing and interpretation. For instance, in many industries, including finance, manufacturing, and logistics, product codes, customer IDs, or other alphanumeric identifiers often follow a specific format that requires leading zeros.
Imagine dealing with a list of zip codes or product codes, where a missing zero can lead to disastrous misinterpretations. Whether preserving data integrity or ensuring uniformity in sorting, leading zeros are the invisible guardians of data accuracy.
Methods for Adding Leading Zeros in SQL
There are several SQL functions and techniques that can be used to add leading zeros to numerical values. Here, we will discuss some commonly used methods:
1. Using the LPAD Function
The LPAD (left pad) function is commonly used in SQL to add leading characters to a string. When applying this function to a numerical value, it is converted to a string and then padded with zeros as required.
Syntax:
LPAD(source_string, total_length, padding_string)
source_string: The original string or number you want to pad.
total_length: The desired total length of the result.
padding_string: The character(s) to use for padding, usually ‘0’ for zeros.
Here is an example of how to use the LPAD function.
SELECT LPAD(’42’, 5, ‘0’) AS PaddedNumber;
In this query, we’re taking the number ’42’ and using LPAD to make it five characters long with leading zeros. The result is ‘00042’.
2. Using the FORMAT Function
Some SQL database systems provide the FORMAT function, which allows for custom formatting of numerical values. You can use this function to add leading zeros to a number. Here is an example:
SELECT FORMAT(column_name, ‘00000’) AS padded_column
FROM table_name;
3. Using Concatenation
You can also employ concatenation to add leading zeros in SQL. By combining the appropriate number of zeros with the original value, you can achieve the desired format. Here is an example:
SELECT CONCAT(‘00000’, column_name) AS padded_column
FROM table_name;
4. Using TO_CHAR Function in Oracle
Oracle provides a functionality called TO_CHAR(number) which enables the addition of leading zeros to a numerical value. This feature generates a string output in the designated format.
SELECT TO_CHAR(9, ‘0000’) AS LEADING_ZEROS FROM dual;
In this example, the number 9 gets converted to a string with four digits, where leading zeros are added to make it ‘0009’.
Padding with Zeros in Different Data Types
Adding leading zeros isn’t one-size-fits-all; it varies depending on your data type. Let’s explore how to pad zeros for different types of data.
Integers
For integers, you can use LPAD as mentioned earlier. Just convert your integer to a string if needed and apply the appropriate function.
SELECT LPAD(CAST(42 AS VARCHAR), 5, ‘0’) AS PaddedNumber;
Strings
When working with strings, it’s often about ensuring a minimum length. Whether it’s a name or description, you can use LPAD to guarantee a consistent character count.
SELECT LPAD(‘SQL Rocks’, 15, ‘ ‘) AS PaddedString;
Dates
You might need to add leading zeros to months or days to keep the format consistent. LPAD is your friend here as well.
SELECT CONCAT(
LPAD(EXTRACT(MONTH FROM current_date), 2, ‘0’), ‘/’,
LPAD(EXTRACT(DAY FROM current_date), 2, ‘0’), ‘/’,
EXTRACT(YEAR FROM current_date)
) AS PaddedDate;
Handling Conditional Padding
There are cases where you only want to add leading zeros under certain conditions. SQL is flexible enough to accommodate such scenarios. Let’s explore an example where we conditionally pad a number only if it’s less than a certain value:
SELECT CASE
WHEN number_column < 100 THEN LPAD(CAST(number_column AS VARCHAR), 3, ‘0’)
ELSE CAST(number_column AS VARCHAR)
END AS PaddedNumber
FROM your_table;
Frequently Asked Questions
Can I add leading zeros to a date in SQL?
Yes, you can add leading zeros to dates in SQL. You can use the LPAD function to ensure that the day or month portion of your date is consistently formatted.
How can I dynamically calculate the required number of leading zeros in SQL?
To dynamically calculate the required number of leading zeros in SQL, you can use the LENGTH function to determine the current length of your data. You can then calculate the additional leading zeros needed to achieve your desired total length.
Conclusion
For sorting, filtering, or maintaining consistency, adding leading zeros can make a big impact. In this article, we’ve explored the LPAD, FORMAT, and CONCAT functions and their use for different data types and conditional padding. Now, armed with this knowledge, it’s time to add those leading zeros and watch your data fall neatly into place!