Creating Tables Safely with SQL | The “CREATE TABLE IF NOT EXISTS” Statement
When working with SQL to create tables, it’s essential to ensure that the table is created only if it doesn’t already exist. This prevents errors and maintains data integrity. The “CREATE TABLE IF NOT EXISTS” statement comes in handy for this purpose.
It is basically the exact opposite of the CREATE OR REPLACE TABLE statement which drops the existing table and replaces it with the newly defined table.
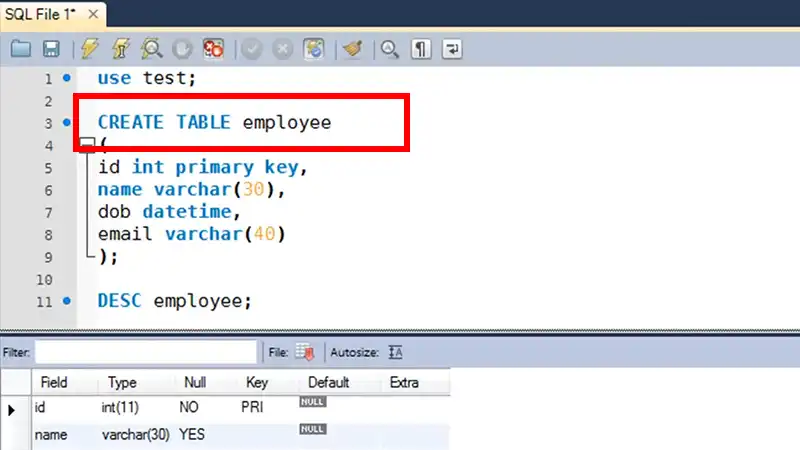
How to Use CREATE TABLE IF NOT EXISTS?
When employing the IF NOT EXISTS clause, the table creation process ensures that a table is only generated if there isn’t already an existing table with the same name. In case a table with the specified name already exists, the default behavior is to trigger a warning.
Syntax and Usage
The syntax is straightforward. You specify the table name and its structure within parentheses. Here’s an example:
CREATE TABLE IF NOT EXISTS customers (
id INT,
name VARCHAR(50),
email VARCHAR(100)
);
In this example, the table “customers” will only be created if it doesn’t already exist. The columns “id,” “name,” and “email” are defined within the parentheses.
Supported RDBMSs
The “CREATE TABLE IF NOT EXISTS” statement is supported by various relational database management systems (RDBMSs) including MySQL, MariaDB, PostgreSQL, and SQLite. However, it’s worth noting that some systems may lack support or have specific implementations.
CREATE TABLE IF NOT EXISTS: SQL Server
SQL Server, for instance, lacks native support for the “CREATE TABLE IF NOT EXISTS” statement. However, we can achieve the same result using alternative methods. One such approach involves utilizing the OBJECT ID() function to check for the existence of the table before creating it.
IF OBJECT_ID(N'dbo.customers', N'U') IS NULL
CREATE TABLE dbo.customers (
id INT,
name VARCHAR(50),
email VARCHAR(100)
);
In this example, we’re checking the existence of the “customers” table within the “dbo” schema. If the table doesn’t exist (as indicated by NULL returned from OBJECT_ID()), the table creation script is executed.
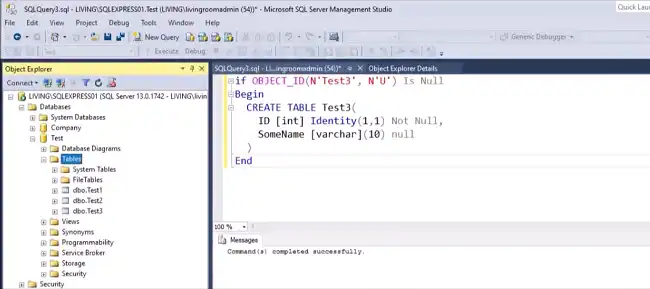
CREATE TABLE IF NOT EXISTS: Oracle
Before Oracle Database 23c, the CREATE TABLE IF NOT EXISTS syntax wasn’t available. In Oracle 21c or earlier versions, an alternative approach is necessary. Here’s a workaround.
DECLARE
table_count NUMBER;
create_statement VARCHAR2(1000);
BEGIN
-- Count the number of tables with the specified name in the HR schema
SELECT COUNT(*) INTO table_count
FROM dba_tables
WHERE owner = 'HR'
AND table_name = 'EMPLOYEES';
-- If no table with the specified name exists, create it
IF(table_count <= 0) THEN
create_statement := '
CREATE TABLE EMPLOYEES (
employee_id NUMBER,
first_name VARCHAR2(50),
last_name VARCHAR2(50),
email VARCHAR2(100),
hire_date DATE
)';
EXECUTE IMMEDIATE create_statement;
END IF;
END;
In this example, we first count the number of tables with the name “EMPLOYEES” in the HR schema. If no table with that name exists, we dynamically generate and execute a CREATE TABLE statement to create the “EMPLOYEES” table with the specified columns. This ensures that the table is only created if it doesn’t already exist in the database.
Frequently Asked Questions
Where use NOT EXISTS vs NOT IN?
The NOT IN operator is utilized to search for data within a finite list of values or a subquery. On the other hand, the NOT EXISTS operator is employed to assess a subquery and yields true if the specified row is absent.
How to create a table if table does not exist in MySQL?
In MySQL, the syntax for using CREATE TABLE IF NOT EXISTS is the same as other SQL options. No alternative approaches are required.
Conclusion
Creating a new table in a database typically involves using the CREATE TABLE statement, which mandates a unique name for the table. While it’s essential to assign distinct names to tables within a database, the IF NOT EXISTS constraint offers an optional feature to check if a table with the same name already exists.