Troubleshooting ‘Attribute Error | Module ‘pandas’ has no Attribute ‘read_sql’
Encountering an ‘Attribute Error’ while working with Python’s Pandas library can be frustrating, especially concerning a commonly used function like ‘read_sql.’ This error typically indicates that the specified attribute or method does not exist within the module or object being referenced. In this article, we’ll delve into the potential causes of this error and explore steps to troubleshoot and resolve it effectively.
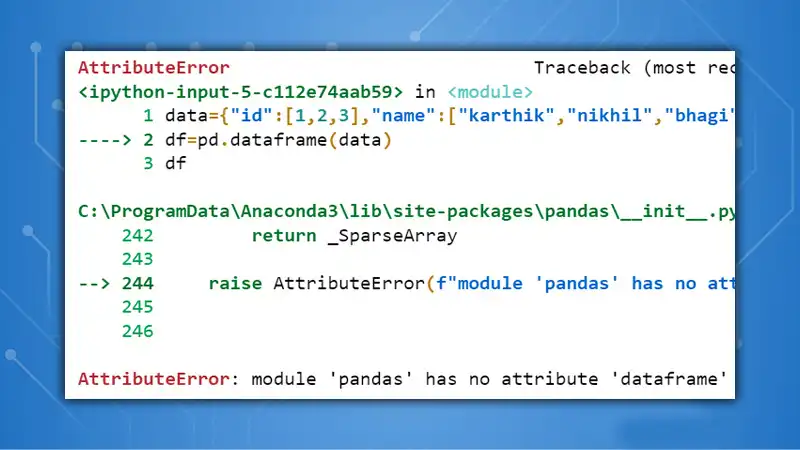
Understanding the Error
The ‘Attribute Error: Module ‘pandas’ has no attribute ‘read_sql” suggests that the Pandas library does not recognize the ‘read_sql’ function. This function is commonly used to read data from SQL databases directly into a data frame, making it a crucial tool for data analysis tasks.
Potential Causes
Several factors could contribute to this error:
Incorrect Pandas Version
Ensure that you’re using a version of Pandas that supports the ‘read_sql’ function. Older versions may lack this functionality, leading to the attribute error.
Installation Issues
If Pandas is not installed correctly or is corrupted, it may fail to recognize certain attributes or methods, including ‘read_sql.’
Misspelling or Typo
Double-check the syntax and spelling of your code to ensure there are no typos or misspelled attribute names.
Troubleshooting Steps
To resolve the ‘Attribute Error: Module ‘pandas’ has no attribute ‘read_sql”:
Check Pandas Version
Upgrade Pandas to the latest version using pip or conda to ensure compatibility with the ‘read_sql’ function.
Reinstall Pandas
If you suspect installation issues, uninstall Pandas using pip or conda and then reinstall it to ensure a clean installation.
Verify Syntax
Review your code and ensure that the syntax for calling the ‘read_sql’ function is correct. The correct syntax is typically ‘pd.read_sql(sql_query, connection_object).’
Example Code
import pandas as pd
from sqlalchemy import create_engine
# Establish a connection to your SQL database
engine = create_engine('sqlite:///example.db')
# Define your SQL query
sql_query = 'SELECT * FROM your_table'
# Use read_sql to fetch data from the database into a DataFrame
try:
df = pd.read_sql(sql_query, engine)
print(df.head())
except AttributeError as e:
print(f"Attribute Error: {e}")
Further Considerations
Documentation
Consult the Pandas documentation for information on the ‘read_sql’ function and its usage.
Community Support
Seek assistance from online forums, such as Stack Overflow, where developers may have encountered similar issues and provided solutions.
Frequently Asked Questions (FAQ)
How can I identify the specific Pandas version that supports the ‘read_sql’ function?
You can check the Pandas documentation or use the command pd.__version__ to display the installed Pandas version.
Are there alternative methods for reading data from SQL databases into Pandas DataFrames?
Yes, besides ‘read_sql,’ Pandas also provides the ‘read_sql_query’ and ‘read_sql_table’ functions, which offer similar functionality for different types of SQL queries.
Can the ‘Attribute Error: Module ‘pandas’ has no attribute ‘read_sql” be caused by missing dependencies?
Yes, ensure that all required dependencies, such as SQLAlchemy for database connections, are properly installed and up-to-date.
Conclusion
The ‘Attribute Error: Module ‘pandas’ has no attribute ‘read_sql” can be resolved by ensuring compatibility with the Pandas version, verifying installation integrity, and reviewing code syntax. By following the troubleshooting steps outlined in this article, developers can effectively address this error and continue leveraging Pandas for efficient data analysis tasks.