How to Get Quarterly Data in SQL Server
Structured Query Language (SQL) is a powerful tool for managing and extracting data from relational databases. One common requirement in data analysis is the need to retrieve information every quarter.
This article will guide you through the process of obtaining quarterly data in SQL Server, providing step-by-step instructions and best practices.
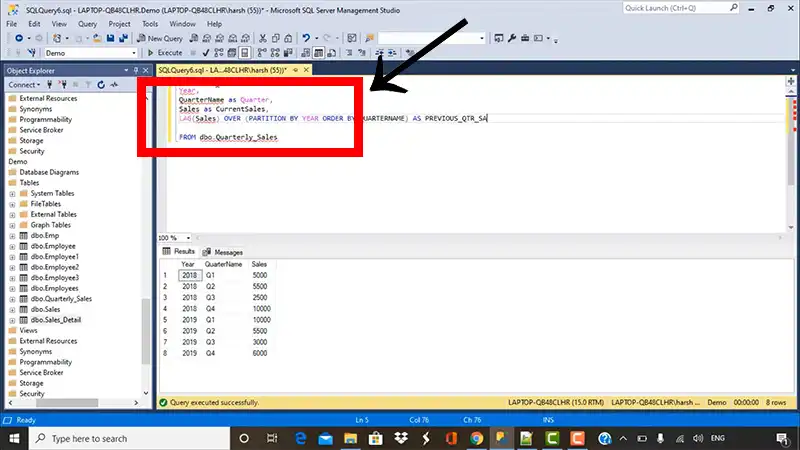
How to Retrieve Quarterly Data
To retrieve the quarterly data, follow the steps below:
Identify the Date Column
Begin by identifying the date column in the table you are working with. This column will be the key to filtering and grouping the data by quarters.
SELECT *
FROM YourTableName
ORDER BY YourDateColumnName;
Filter Data for a Specific Quarter
To retrieve data for a specific quarter, use the DATEPART function to extract the quarter from the date column.
SELECT *
FROM YourTableName
WHERE DATEPART(QUARTER, YourDateColumnName) = 1; — Change 1 to the desired quarter (1-4)
Filter Data for a Specific Year and Quarter
To narrow down the data for a particular year and quarter, use both the DATEPART function and the year part.
SELECT *
FROM YourTableName
WHERE DATEPART(YEAR, YourDateColumnName) = 2023
AND DATEPART(QUARTER, YourDateColumnName) = 1; — Change year and quarter as needed
Aggregate Data for Each Quarter
If you want to retrieve aggregated data for each quarter, use the GROUP BY clause along with the SUM, AVG, or other aggregate functions.
SELECT
DATEPART(YEAR, YourDateColumnName) AS Year,
DATEPART(QUARTER, YourDateColumnName) AS Quarter,
SUM(YourNumericColumn) AS TotalSales
FROM YourTableName
GROUP BY
DATEPART(YEAR, YourDateColumnName),
DATEPART(QUARTER, YourDateColumnName);
Indexing
Ensure that the date column is indexed to optimize query performance, especially when dealing with large datasets. This can significantly speed up the retrieval process.
CREATE INDEX IX_YourDateColumn ON YourTableName (YourDateColumnName);
Use Views for Convenience
Consider creating views that encapsulate the logic for quarterly data retrieval. This can simplify queries and make them more maintainable.
CREATE VIEW QuarterlyDataView AS
SELECT *
FROM YourTableName
WHERE DATEPART(QUARTER, YourDateColumnName) = 1;
Frequently Asked Questions
Why is it important to retrieve quarterly data in SQL Server?
Retrieving quarterly data is crucial for various analytical and reporting tasks. Many businesses and organizations operate on a quarterly reporting schedule, making it necessary to extract and analyze data at a granular level to gain insights into performance, trends, and key metrics over specific quarters.
What SQL functions can I use to filter data by quarters in SQL Server?
To filter data by quarters, you can use the DATEPART function in SQL Server. Specifically, DATEPART(QUARTER, YourDateColumn) extracts the quarter from a given date column. This function allows you to filter, group, and aggregate data based on quarterly intervals.
Are there performance considerations when retrieving quarterly data in SQL Server?
Yes, performance considerations are important, especially when dealing with large datasets. Indexing the date column, using appropriate WHERE clauses, and optimizing queries can significantly enhance the speed of retrieving quarterly data. It’s recommended to assess and optimize query performance for efficiency.
Conclusion
Retrieving quarterly data in SQL Server involves leveraging date functions and filtering techniques. Remember to optimize your queries, consider indexing strategies, and use views for improved maintainability. With these practices, you’ll be well-equipped to handle quarterly data retrieval in SQL Server effectively.