AWS S3 Check if File Exists | Simplified
Ensuring the presence of a file in your Amazon S3 bucket is essential for effective data management. In AWS S3, you can check if a file exists using the AWS Command Line Interface (CLI), AWS SDKs (such as Boto3 for Python), or through the AWS Management Console.
In this article, we explained two of these practical approaches to swiftly check file existence. Whether you favor CLI commands or Python scripting with Boto3, we’ve got you covered. So, let’s dive right in.
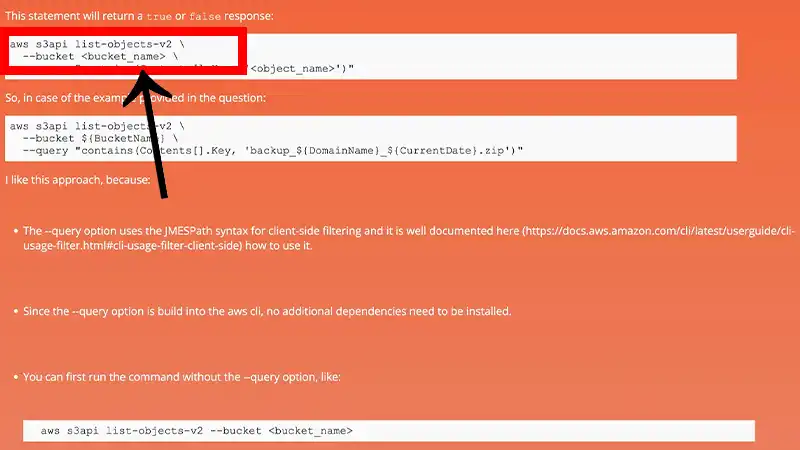
How to Check If a File Exists in S3 Bucket Using AWS CLI
Below is a demonstration of how you can use AWS CLI with two different commands to check S3 file existence.
Method 1: Using the aws s3 ls Command
You can use the aws s3 ls command to list the contents of an S3 bucket and then check if the file exists in the list. Here’s an example:
aws s3 ls s3://your-bucket-name/your-file-key
Replace your-bucket-name with the name of your S3 bucket and your-file-key with the key or path of the file within the bucket. If the file exists, you will see information about the file; otherwise, there will be no output.
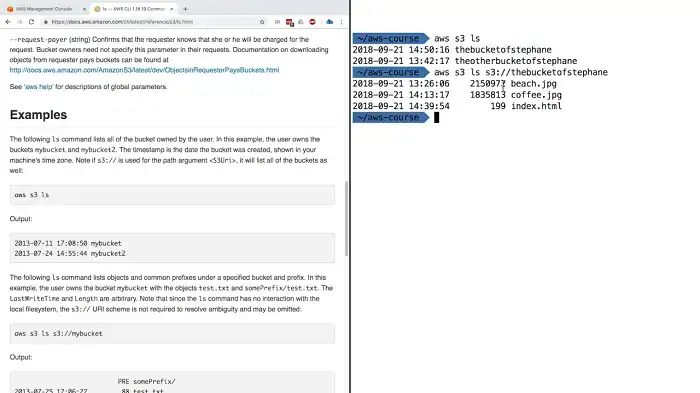
Method 2: Using s3api head-object
To check if a file exists in Amazon S3 using the AWS Command Line Interface (AWS CLI), you can use the s3api command along with the head-object operation. Here’s an example:
aws s3api head-object –bucket YOUR_BUCKET_NAME –key YOUR_FILE_KEY
Replace YOUR_BUCKET_NAME with the name of your S3 bucket and YOUR_FILE_KEY with the key (path) of the file you want to check. If the file exists, the command will return metadata information about the object. If the file doesn’t exist, you’ll get an error.
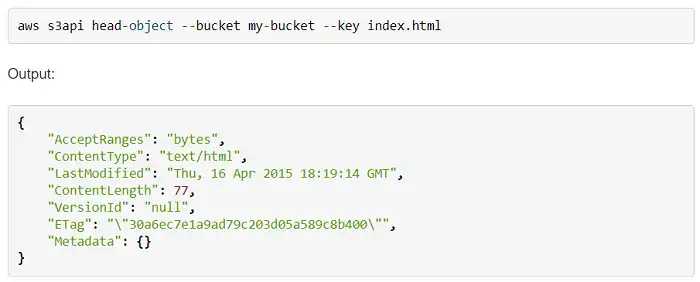
Running s3api head-object With Conditions
Here’s an example with a conditional statement in a shell script:
#!/bin/bash
BUCKET_NAME="YOUR_BUCKET_NAME"
FILE_KEY="YOUR_FILE_KEY"
# Check if the file exists
if aws s3api head-object --bucket $BUCKET_NAME --key $FILE_KEY 2>/dev/null; then
echo "File exists."
else
echo "File does not exist."
fi
In this script, replace YOUR_BUCKET_NAME and YOUR_FILE_KEY with your actual bucket name and file key. The 2>/dev/null part is used to redirect error output to null, so you won’t see error messages on the console if the file doesn’t exist.
How to Check If a File Exists in S3 Bucket Using Boto3
Boto3 is the official AWS SDK for Python, and it provides a convenient way to interact with AWS services, including S3. To check if a file exists in an S3 bucket using Boto3, follow these steps:
1. Install Boto3:
pip install boto3
2. Use the following Python code snippet to check if a file exists:
import boto3
def check_file_exists(bucket_name, key):
s3 = boto3.client('s3')
try:
s3.head_object(Bucket=bucket_name, Key=key)
print(f"The file with key '{key}' exists in the bucket '{bucket_name}'.")
return True
except Exception as e:
if e.response['Error']['Code'] == '404':
print(f"The file with key '{key}' does not exist in the bucket '{bucket_name}'.")
return False
else:
# Handle other exceptions as needed
print(f"An error occurred: {e}")
return False
# Example usage:
bucket_name = 'your_bucket_name'
file_key = 'path/to/your/file.txt'
check_file_exists(bucket_name, file_key)
Replace ‘your_bucket_name’ and ‘path/to/your/file.txt’ with your actual S3 bucket name and file key. If the file exists, it will print a message indicating its existence; otherwise, it will handle the exception and print a message stating that the file does not exist.
Related Questions
- What is the difference between s3 ls and s3api head-object for checking file existence?
s3 ls is used to list objects in a bucket, providing a way to check if a file exists. On the other hand, s3api head-object directly checks the existence of a specific object without listing all objects in a bucket.
- Can I check if a file exists in S3 without affecting my data transfer costs?
Yes, checking file existence using methods like s3 ls (AWS CLI) or head_object (Boto3) allows you to validate without incurring additional data transfer costs, as it doesn’t involve downloading the actual file.
Conclusion
For large-scale applications, it’s recommended to use the s3api head-object or Boto3’s head_object method as they offer a more direct and efficient way to check file existence without listing all objects in a bucket. Just keep in mind that AWS CLI and the described methods need to be installed and configured with the necessary credentials for accessing your S3 bucket.