Writing to the File System in an Azure Web App
Azure Web Apps is a popular service that allows developers to quickly build, deploy, and scale web applications on Microsoft Azure. One common requirement for web applications is the ability to write to the file system. However, when working with Azure Web Apps, there are specific considerations and best practices to follow.
This article will guide you through the process of writing to the file system in an Azure Web App, covering key concepts, implementation details, and best practices.
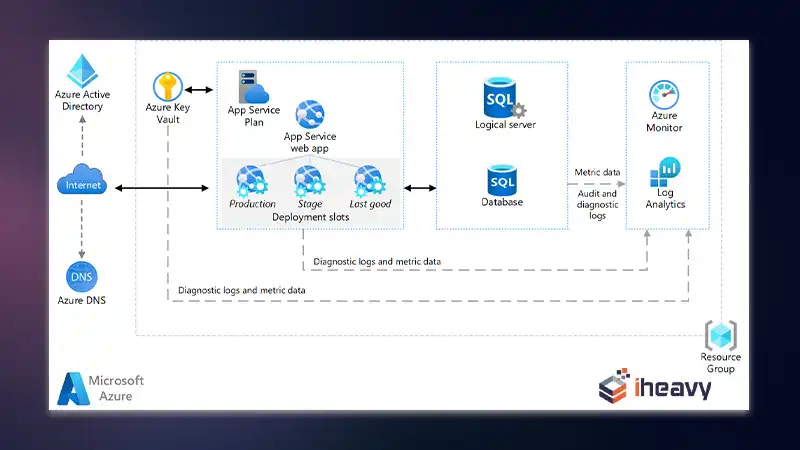
Understanding File System in Azure Web Apps
In Azure Web Apps, the file system is structured to support the needs of web applications while maintaining security and scalability. There are two primary storage areas:
Persistent Storage
The D:\home directory is the persistent storage available to your web app. Files stored here are retained across restarts and deployments.
Temporary Storage
The D:\local directory is the temporary storage, which is reset upon app restart and redeployment. Data here is ephemeral and should not be used for long-term storage.
Writing to the File System
Step-by-Step Guide
Setting Up Your Azure Web App
First, ensure that you have an Azure Web App set up. You can create a new web app through the Azure portal, Azure CLI, or other deployment methods.
Accessing Persistent Storage
When writing files to the persistent storage, use the D:\home directory. This ensures that your files remain available across app restarts and deployments.
// Example in C#
using System.IO;
public void WriteToPersistentStorage()
{
string path = @"D:\home\site\wwwroot\example.txt";
string content = "Hello, Azure!";
using (StreamWriter writer = new StreamWriter(path))
{
writer.WriteLine(content);
}
}
Accessing Temporary Storage
For temporary data that does not need to persist, use the D:\local directory. Remember that data in this directory will be lost upon app restart or redeployment.
// Example in C#
using System.IO;
public void WriteToTemporaryStorage()
{
string path = @"D:\local\Temp\example.txt";
string content = "This is temporary data.";
using (StreamWriter writer = new StreamWriter(path))
{
writer.WriteLine(content);
}
}
Best Practices
Use Persistent Storage for Critical Data
Always write critical data to the D:\home directory to ensure it persists across app restarts.
Monitor Storage Quotas
Be mindful of the storage quotas for your Azure Web App plan to avoid running out of space.
Consider Azure Storage for Large Files
For large files or extensive data storage needs, consider using Azure Blob Storage instead of local file system storage.
Implement Error Handling
Include proper error handling to manage situations where the file system may not be available or writable.
Frequently Asked Questions
Can I write to any directory in an Azure Web App?
No, you should only write to the designated directories (D:\home for persistent storage and D:\local for temporary storage). Writing to other directories may not be supported and could lead to errors or data loss.
How can I check the available storage space for my Azure Web App?
You can monitor storage usage through the Azure portal. Navigate to your web app and check the “Disk Usage” under the “Diagnose and solve problems” section.
What happens to the data in the D:\local directory after a restart?
The D:\local directory is temporary storage, and its contents are cleared upon app restart or redeployment. Use this directory only for data that does not need to persist.
Is it possible to increase the storage capacity for my Azure Web App?
Yes, you can scale up your Azure Web App plan to a higher tier that offers more storage capacity. This can be done through the Azure portal.
Should I use the file system for storing large amounts of data?
For storing large amounts of data, it is recommended to use Azure Blob Storage instead of the local file system to ensure scalability and reliability.
Conclusion
Writing to the file system in an Azure Web App is straightforward once you understand the designated storage areas and best practices. By using the D:\home directory for persistent storage and the D:\local directory for temporary storage, you can effectively manage your application’s file storage needs. Always consider the specific requirements of your application and use Azure’s broader storage solutions when necessary for optimal performance and scalability.